This website requires JavaScript.
- Learning Angular
- Architecture
- Displaying Data
- Dependency Injection
- Template Syntax
- Introduction
- 0. The Starter App
- 1. The Hero Editor
- 2. Master/Detail
- 3. Multiple Components
- 4. Services
- Attribute Directives
- Component Styles
- Hierarchical Injectors
- HTTP Client
- Lifecycle Hooks
- Structural Directives
- Components Gallery
- Cheat Sheet
- Community and Support
- API Documentation
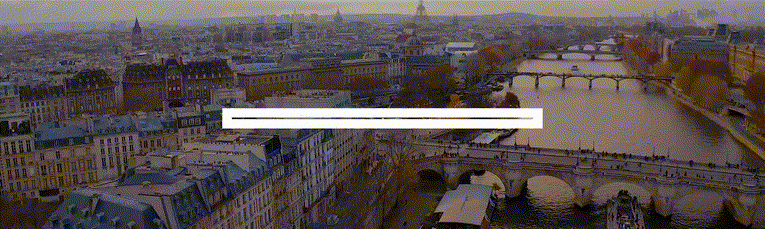
On this page
The end game.
new_releases We're currently in the progress of migrating this site from Angular version 5.3.1 to 7.0.0. Package versions might be out-of-date or inconsistent! Visit the repo for more info.
Tutorial: Tour of Heroes 6.0
The grand plan for this tutorial is to build an app that helps a staffing agency manage its stable of heroes.
The Tour of Heroes app covers the core fundamentals of Angular. You’ll build a basic app that has many of the features you’d expect to find in a full-blown, data-driven app: acquiring and displaying a list of heroes, editing a selected hero’s detail, and navigating among different views of heroic data. You’ll learn the following:
- Use built-in directives to show and hide elements and display lists of hero data.
- Create components to display hero details and show an array of heroes.
- Use one-way data binding for read-only data.
- Add editable fields to update a model with two-way data binding.
- Bind component methods to user events, like keystrokes and clicks.
- Enable users to select a hero from a master list and edit that hero in the details view.
- Format data with pipes.
- Create a shared service to assemble the heroes.
- Use routing to navigate among different views and their components.
You’ll learn enough core Angular to get started and gain confidence that Angular can do whatever you need it to do. You’ll cover a lot of ground at an introductory level, and you’ll find many links to pages with greater depth.
When you’re done with this tutorial, the app will look like this live example ( view source ) .
Here’s a visual idea of where this tutorial leads, beginning with the “Dashboard” view and the most heroic heroes:
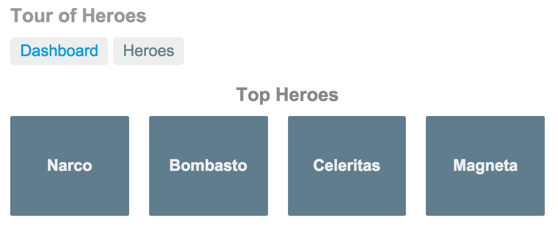
You can click the two links above the dashboard (“Dashboard” and “Heroes”) to navigate between this Dashboard view and a Heroes view.
If you click the dashboard hero “Magneta,” the router opens a “Hero Details” view where you can change the hero’s name.
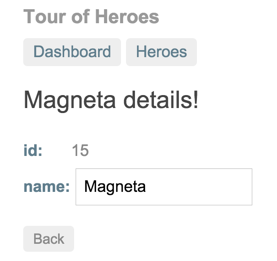
Clicking the “Back” button returns you to the Dashboard. Links at the top take you to either of the main views. If you click “Heroes,” the app displays the “Heroes” master list view.
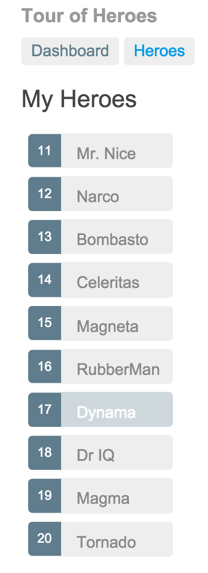
When you click a different hero name, the read-only mini detail beneath the list reflects the new choice.
You can click the “View Details” button to drill into the editable details of the selected hero.
The following diagram captures all of the navigation options.
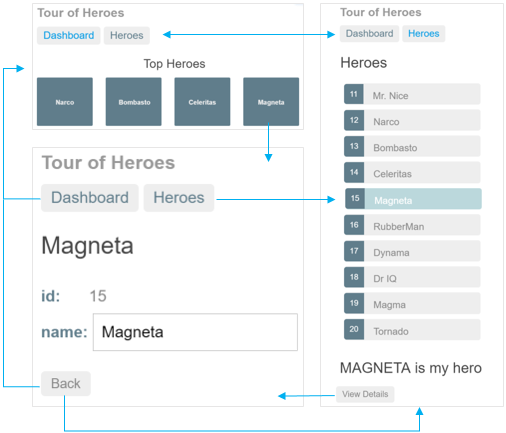
Here’s the app in action:
You’ll build the Tour of Heroes app, step by step. Each step is motivated with a requirement that you’ve likely met in many apps. Everything has a reason.
Along the way, you’ll become familiar with many of the core fundamentals of Angular.
Angular Tour of Heroes
The tour of heroes tutorial covers the fundamentals of angular., in this tutorial you will build an app that helps a staffing agency manage its stable of heroes..
This basic app has many of the features you'd expect to find in a data-driven application. It acquires and displays a list of heroes, edits a selected hero's detail, and navigates among different views of heroic data.
This project reinforce skills such as:
- Use built-in Angular directives to show and hide elements and display lists of hero data.
- Create Angular components to display hero details and show an array of heroes.
- Use one-way data binding for read-only data.
- Add editable fields to update a model with two-way data binding.
- Bind component methods to user events, like keystrokes and clicks.
- Enable users to select a hero from a master list and edit that hero in the details view.
- Format data with pipes.
- Create a shared service to assemble the heroes.
- Use routing to navigate among different views and their components.
Usage Guide
- You need to install the dependencies:
- You can use 'compodoc run' to start building your documentation:
Configuration
- Change compodoc theme:
- Add assets folder to insert images into Markdown files:
Dependencies:
Result-matching " ", no results matching " ".
Tutorial: Tour of Heroes
The Tour of Heroes tutorial takes us through the steps of creating an Angular application in TypeScript.
This page is not yet available in JavaScript. We recommend reading it in TypeScript .

deep dive into code
Angular Tutorial - Tour of Heroes - Part 6 (Add navigation with routing)
Introduction, routermodule.forroot(), add routeroutlet, add a navigation link using routerlink, add the dashboard route, add a default route, add dashboard link to the shell, delete hero details from heroescomponent, add a hero detail route, dashboardcomponent hero links, heroescomponent hero links, remove dead code - optional, extract the id route parameter, add heroservice.gethero(), find the way back.
This tutorial recaps the official documentation at https://angular.io/tutorial/tour-of-heroes/toh-pt5 .
The Tour of Heroes application has new requirements: Add a Dashboard view Add the ability to navigate between the Heroes and Dashboard views When users click a hero name in either view, navigate to a detail view of the selected hero When users click a deep link in an email, open the detail view for a particular hero
When you’re done, users can navigate the application like this:
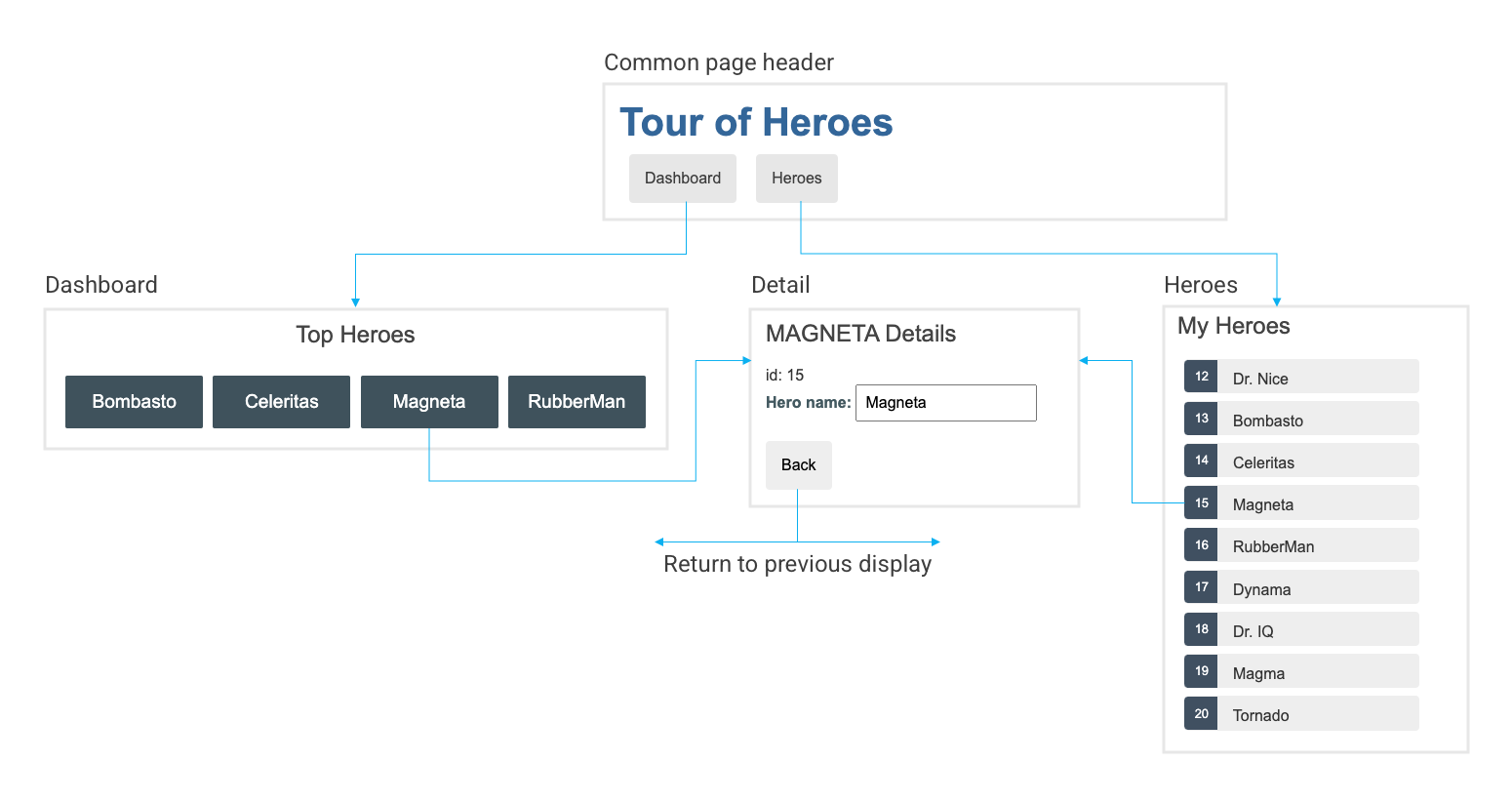
(original image from https://angular.io/generated/images/guide/toh/nav-diagram.png )
Add the AppRoutingModule
In Angular, the best practice is to load and configure the router in a separate, top-level module. The router is dedicated to routing (URLs to target views) and imported by the root AppModule .
By convention, the module class name is AppRoutingModule and it belongs in the app-routing.module.ts in the src/app directory.
Run ng generate to create the application routing module.
- --flat : Puts the file in src/app instead of its own directory.
- --module=app : Tells ng generate to register it in the imports array of the AppModule .
File src/app/app.module.ts :
The file src/app/app-routing.module.ts that ng generate created looks like this:
Replace it with the following:
First, the app-routing.module.ts file imports RouterModule and Routes so the application can have routing capability. The next import, HeroesComponent , gives the Router somewhere to go once you configure the routes.
Notice that the CommonModule references and declarations array are unnecessary, so are no longer part of AppRoutingModule . The following sections explain the rest of the AppRoutingModule in more detail.
The next part of the file is where you configure your routes. Routes tell the Router which view to display when a user clicks a link or pastes a URL into the browser address bar.
Since app-routing.module.ts already imports HeroesComponent , you can use it in the routes array:
File src/app/app-routing.module.ts :
A typical Angular Route (see https://angular.io/api/router/Route ) has two properties:
- path : A string that matches the URL in the browser address bar.
- component : The component that the router should create when navigating to this route.
This tells the router to match that URL to path: 'heroes' and display the HeroesComponent when the URL is something like http://localhost:4200/heroes .
The @NgModule metadata initializes the router and starts it listening for browser location changes.
The following line adds the RouterModule to the AppRoutingModule imports array and configures it with the routes in one step by calling RouterModule.forRoot() .
Note: The method is called forRoot() because you configure the router at the application’s root level. The forRoot() method supplies the service providers and directives needed for routing, and performs the initial navigation based on the current browser URL.
Next, AppRoutingModule exports RouterModule to be available throughout the application.
Open the AppComponent template and replace the <app-heroes> element with a <router-outlet> element.
File src/app/app.component.html :
The AppComponent template no longer needs <app-heroes> because the application only displays the HeroesComponent when the user navigates to it.
The <router-outlet> tells the router where to display (“embed”) routed views.
The RouterOutlet is one of the router directives that became available to the AppComponent because AppModule imports AppRoutingModule which exported RouterModule . The ng generate command you ran at the start of this tutorial added this import because of the --module=app flag. If you didn’t use the ng generate command to create app-routing.module.ts , import AppRoutingModule into app.module.ts and add it to the imports array of the NgModule .
If you’re not still serving your application, run ng serve to see your application in the browser under http://localhost:4200/ .
The browser should refresh and display the application title but not the list of heroes.
Look at the browser’s address bar. The URL ends in / . The route path to HeroesComponent is /heroes .
Append /heroes to the URL in the browser address bar: http://localhost:4200/heroes .
You should see the familiar heroes overview/detail view.
Remove /heroes from the URL in the browser address bar. The browser should refresh and display the application title but not the list of heroes.
Ideally, users should be able to click a link to navigate rather than pasting a route URL into the address bar.
Add a <nav> element and, within that, an anchor element that, when clicked, triggers navigation to the HeroesComponent . The revised AppComponent template looks like this:
A routerLink attribute is set to “/heroes”, the string that the router matches to the route to HeroesComponent . The routerLink is the selector for the RouterLink directive that turns user clicks into router navigations. It’s another of the public directives in the RouterModule .
The browser refreshes and displays the application title and heroes link, but not the heroes list.
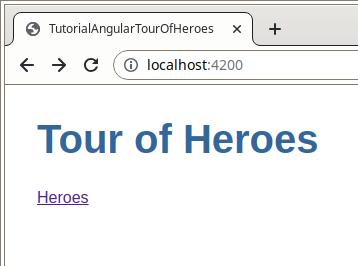
Click the link. The address bar updates to /heroes and the list of heroes appears.
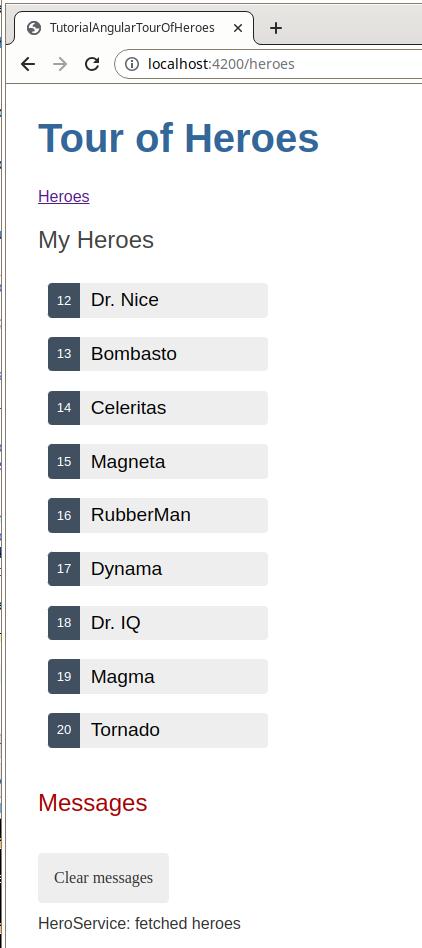
Make this and future navigation links look better by adding private CSS styles to src/app/app.component.css :
Add a dashboard view
Routing makes more sense when your application has more than one view, yet the Tour of Heroes application has only the heroes view.
To add a DashboardComponent , run ng generate as shown here:
ng generate creates the files for the DashboardComponent and declares it in AppModule .
Replace the default content in these files as shown here:
File src/app/dashboard/dashboard.component.html :
The template presents a grid of hero name links.
- The *ngFor repeater creates as many links as are in the component’s heroes array.
- The links are styled as colored blocks by the dashboard.component.css .
- The links don’t go anywhere yet.
File src/app/dashboard/dashboard.component.ts :
The class is like the HeroesComponent class.
- It defines a heroes array property
- The constructor expects Angular to inject the HeroService into a private heroService property
- The ngOnInit() lifecycle hook calls getHeroes()
- This getHeroes() returns the sliced list of heroes at positions 1 and 5, returning only Heroes two, three, four, and five.
File src/app/dashboard/dashboard.component.css :
To navigate to the dashboard, the router needs an appropriate route.
Import the DashboardComponent in the app-routing.module.ts file and add a route to the routes array that matches the path dashboard to the DashboardComponent .
When the application starts, the browser’s address bar points to the web site’s root ( '' or / ). That doesn’t match any existing route so the router doesn’t navigate anywhere. The space below the <router-outlet> is blank.
To make the application navigate to the dashboard automatically, add the following route to the routes array.
This route redirects a URL that fully matches the empty path to the route whose path is ‘/dashboard’.
After the browser refreshes, the router loads the DashboardComponent and the browser address bar shows the /dashboard URL.
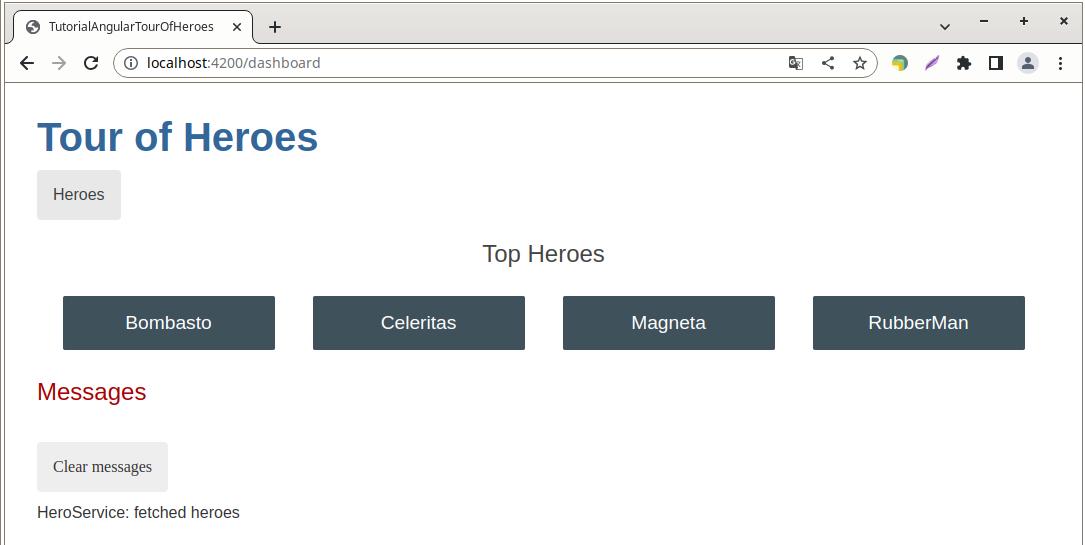
The user should be able to navigate between the DashboardComponent and the HeroesComponent by clicking links in the navigation area near the top of the page.
Add a dashboard navigation link to the AppComponent shell template, just above the Heroes link.
After the browser refreshes you can navigate freely between the two views by clicking the links.
Navigating to hero details
The HeroDetailComponent displays details of a selected hero. At the moment the HeroDetailComponent is only visible at the bottom of the HeroesComponent .
The user should be able to get to these details in three ways.
- By clicking a hero in the dashboard.
- By clicking a hero in the heroes list.
- By pasting a “deep link” URL into the browser address bar that identifies the hero to display.
This section enables navigation to the HeroDetailComponent and liberates it from the HeroesComponent .
When the user clicks a hero in HeroesComponent , the application should navigate to the HeroDetailComponent , replacing the heroes list view with the hero detail view. The heroes list view should no longer show hero details as it does now.
Open the src/app/heroes/heroes.component.html and delete the <app-hero-detail> element from the bottom.
Clicking a hero item now does nothing. You can fix that after you enable routing to the HeroDetailComponent .
An URL like ~/detail/11 would be a good URL for navigating to the Hero Detail view of the hero whose id is 11.
Open file src/app/app-routing.module.ts and
- import HeroDetailComponent
- add a parameterized route to the routes array (and we sorted it alphabetically for better overview) that matches the path pattern to the hero detail view
The colon : character in the path indicates that :id is a placeholder for a specific hero id .
At this point, all application routes are in place.
The DashboardComponent hero links do nothing at the moment.
Now that the router has a route to HeroDetailComponent , fix the dashboard hero links to navigate using the parameterized dashboard route.
You’re using Angular interpolation binding (see https://angular.io/guide/interpolation ) within the *ngFor repeater (see https://angular.io/api/common/NgFor ) to insert the current iteration’s hero.id into each routerLink .
The hero items in the HeroesComponent are <li> elements whose click events are bound to the component’s onSelect() method.
Actual File src/app/heroes/heroes.component.html :
Remove the inner HTML of <li> . Wrap the badge and name in an anchor <a> element. Add a routerLink attribute to the anchor that’s the same as in the dashboard template.
New File src/app/heroes/heroes.component.html :
Be sure to fix the private style sheet in src/app/heroes/heroes.component.css to make the list look as it did before:
While the HeroesComponent class still works, the onSelect() method and selectedHero property are no longer used.
It’s nice to tidy things up for your future self. Here’s the class after pruning away the dead code.
File src/app/heroes/heroes.component.ts :
Routable HeroDetailComponent
The parent HeroesComponent used to set the HeroDetailComponent.hero property and the HeroDetailComponent displayed the hero.
HeroesComponent doesn’t do that anymore. Now the router creates the HeroDetailComponent in response to a URL such as ~/detail/12 .
The HeroDetailComponent needs a new way to get the hero to display. This section explains the following:
- Get the route that created it
- Extract the id from the route
- Get the hero with that id from the server using the HeroService
- Add the imports for ActivatedRoute (see https://angular.io/api/router/ActivatedRoute ), Location (see https://angular.io/api/common/Location ) and HeroService
- Inject the ActivatedRoute , HeroService , and Location services into the constructor, saving their values in private fields
File src/app/hero-detail/hero-detail.component.ts :
The ActivatedRoute holds information about the route to this instance of the HeroDetailComponent . This component is interested in the route’s parameters extracted from the URL. The “id” parameter is the id of the hero to display.
The HeroService gets hero data from the remote server and this component uses it to get the hero-to-display.
The Location is an Angular service for interacting with the browser. This service lets you navigate back to the previous view.
- Add implements OnInit and add import for OnInit .
- Change hero property : remove @Input() and change line to hero: Hero | undefined; , remove Input import
- In the ngOnInit() lifecycle hook call getHero() and define it as follows.
File src/app/hero-detail/hero-detail.component.ts
The route.snapshot is a static image of the route information shortly after the component was created.
The paramMap is a dictionary of route parameter values extracted from the URL. The “id” key returns the id of the hero to fetch.
Route parameters are always strings. The JavaScript Number function converts the string to a number, which is what a hero id should be.
The browser refreshes and the application crashes with a compiler error. HeroService doesn’t have a getHero() method. Add it now.
Open HeroService and add the following getHero() method with the id after the getHeroes() method:
File src/app/hero.service.ts :
IMPORTANT: The backtick ( ` ) characters define a JavaScript template literal (see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Template_literals ) for embedding the id .
Like getHeroes() , getHero() has an asynchronous signature. It returns a mock hero as an Observable , using the RxJS of() function.
You can rewrite getHero() as a real Http request without having to change the HeroDetailComponent that calls it (see next tutorial part).
The browser refreshes and the application is working again. You can click a hero in the dashboard or in the heroes list and navigate to that hero’s detail view.
If you paste http://localhost:4200/detail/12 in the browser address bar, the router navigates to the detail view for the hero with id: 12 , “Dr Nice”.
By clicking the browser’s back button, you can go back to the previous page. This could be the hero list or dashboard view, depending upon which sent you to the detail view.
It would be nice to have a button on the HeroDetail view that can do that.
Add a go back button to the bottom of the component template and bind it to the component’s goBack() method.
File src/app/hero-detail/hero-detail.component.html :
Add a goBack() method to the component class that navigates backward one step in the browser’s history stack using the Location service that you used to inject.
Refresh the browser and start clicking. Users can now navigate around the application using the new buttons.
The details look better when you add the private CSS styles to src/app/hero-detail/hero-detail.component.css :
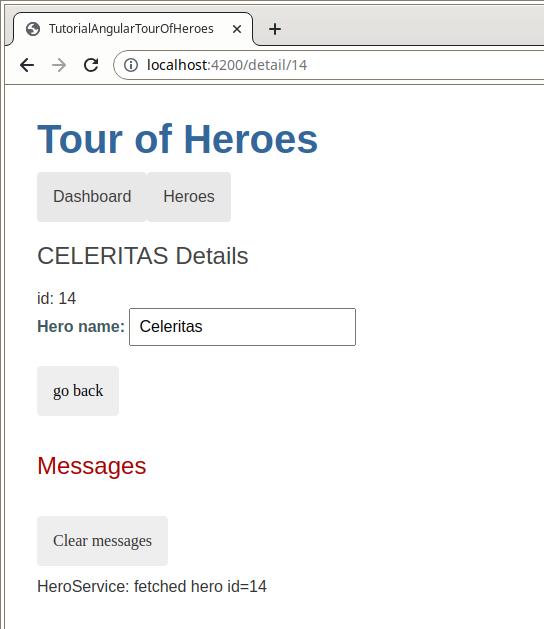
Previous ⏪ Angular Tutorial - Tour of Heroes - Part 5 (Add services)
Next Angular Tutorial - Tour of Heroes - Part 7 (Get data from a server) ⏩
Tour of Heroes app and tutorial
In this tutorial, you build your own app from the ground up, providing experience with the typical development process, as well as an introduction to basic app-design concepts, tools, and terminology.
If you're completely new to Angular, you might want to try the Try it now quick-start app first. It is based on a ready-made partially-completed project, which you can examine and modify in the StackBlitz interactive development environment, where you can see the results in real time.
The "Try it" tutorial covers the same major topics—components, template syntax, routing, services, and accessing data via HTTP—in a condensed format, following the most current best practices.
This Tour of Heroes tutorial shows you how to set up your local development environment and develop an app using the Angular CLI tool , and provides an introduction to the fundamentals of Angular.
The Tour of Heroes app that you build helps a staffing agency manage its stable of heroes. The app has many of the features you'd expect to find in any data-driven application. The finished app acquires and displays a list of heroes, edits a selected hero's detail, and navigates among different views of heroic data.
You will find references to and expansions of this app domain in many of the examples used throughout the Angular documentation, but you don't necessarily need to work through this tutorial to understand those examples.
By the end of this tutorial you will be able to do the following:
- Use built-in Angular directives to show and hide elements and display lists of hero data.
- Create Angular components to display hero details and show an array of heroes.
- Use one-way data binding for read-only data.
- Add editable fields to update a model with two-way data binding.
- Bind component methods to user events, like keystrokes and clicks.
- Enable users to select a hero from a master list and edit that hero in the details view.
- Format data with pipes .
- Create a shared service to assemble the heroes.
- Use routing to navigate among different views and their components.
You'll learn enough Angular to get started and gain confidence that Angular can do whatever you need it to do.
After completing all tutorial steps, the final app will look like this: .
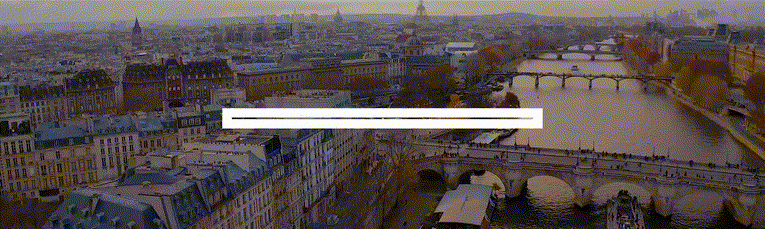
What you'll build
Here's a visual idea of where this tutorial leads, beginning with the "Dashboard" view and the most heroic heroes:
You can click the two links above the dashboard ("Dashboard" and "Heroes") to navigate between this Dashboard view and a Heroes view.
If you click the dashboard hero "Magneta," the router opens a "Hero Details" view where you can change the hero's name.
Clicking the "Back" button returns you to the Dashboard. Links at the top take you to either of the main views. If you click "Heroes," the app displays the "Heroes" master list view.
When you click a different hero name, the read-only mini detail beneath the list reflects the new choice.
You can click the "View Details" button to drill into the editable details of the selected hero.
The following diagram captures all of the navigation options.
Here's the app in action:
© 2010–2020 Google, Inc. Licensed under the Creative Commons Attribution License 4.0. https://v10.angular.io/tutorial
tour-of-heroes-angular-example
The Tour of Heroes tutorial provides an introduction to the fundamentals of Angular. Details at https://angular.io/tutorial
Angular_Tour-Of-Heroes
Created with CodeSandbox
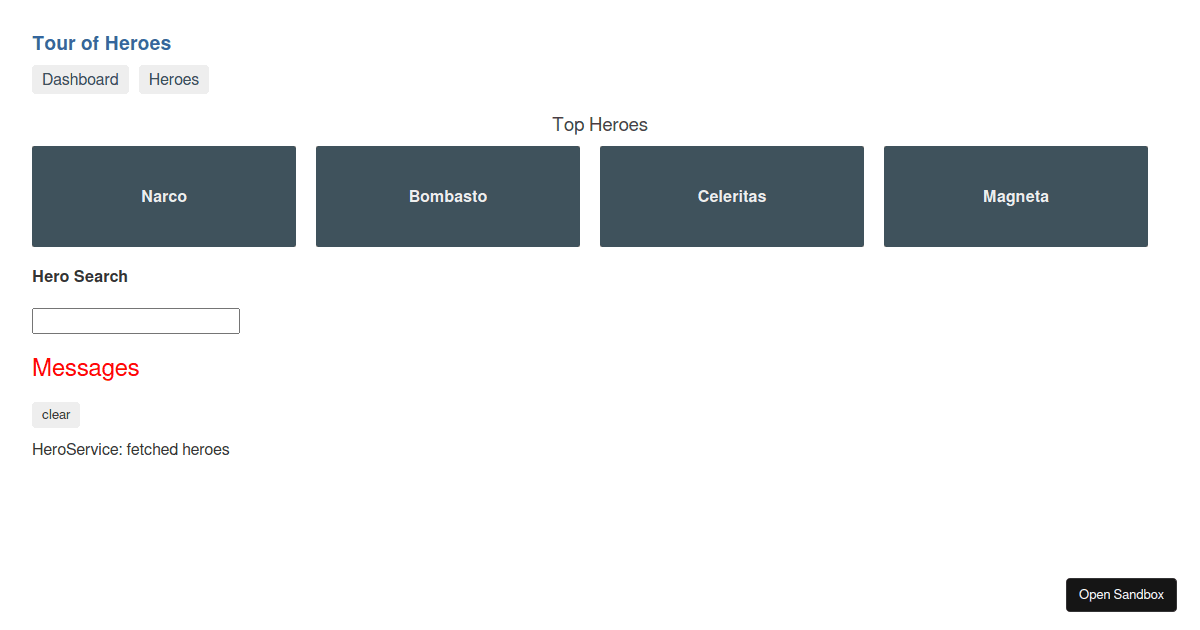
Template type: angular-cli
This sandbox is a live running version of https://www.github.com/Ojage/Angular_Tour-Of-Heroes/tree/main/
Dependencies
- @angular/animations: 8.2.14
- @angular/common: 8.2.14
- @angular/compiler: 8.2.14
- @angular/core: 8.2.14
- @angular/forms: 8.2.14
- @angular/platform-browser: 8.2.14
- @angular/platform-browser-dynamic: 8.2.14
- @angular/router: 8.2.14
- angular-in-memory-web-api: 0.8.0
- core-js: 3.4.2
- jasmine-core: 3.5.0
- jasmine-marbles: 0.6.0
- rxjs: 6.5.3
- tslib: 1.10.0
- web-animations-js: 2.3.2
- zone.js: 0.10.2
Dev Dependencies
- @angular/cli: 1.6.6
- @angular/compiler-cli: ^5.2.0
- @angular/language-service: ^5.2.0
- @types/core-js: 0.9.46
- @types/jasmine: ~2.8.3
- @types/jasminewd2: ~2.0.2
- @types/node: ~6.0.60
- codelyzer: ^4.0.1
- jasmine-core: ~2.8.0
- jasmine-spec-reporter: ~4.2.1
- karma: ~2.0.0
- karma-chrome-launcher: ~2.2.0
- karma-coverage-istanbul-reporter: ^1.2.1
- karma-jasmine: ~1.1.0
- karma-jasmine-html-reporter: ^0.2.2
- protractor: ~5.1.2
- ts-node: ~4.1.0
- tslint: ~5.9.1
- typescript: ~2.5.3
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
roma-hrynko/angular-tour-of-heroes
Folders and files, repository files navigation, angulartourofheroes.
This project was generated with Angular CLI version 17.3.6.
Development server
Run ng serve for a dev server. Navigate to http://localhost:4200/ . The application will automatically reload if you change any of the source files.
Code scaffolding
Run ng generate component component-name to generate a new component. You can also use ng generate directive|pipe|service|class|guard|interface|enum|module .
Run ng build to build the project. The build artifacts will be stored in the dist/ directory.
Running unit tests
Run ng test to execute the unit tests via Karma .
Running end-to-end tests
Run ng e2e to execute the end-to-end tests via a platform of your choice. To use this command, you need to first add a package that implements end-to-end testing capabilities.
Further help
To get more help on the Angular CLI use ng help or go check out the Angular CLI Overview and Command Reference page.
- TypeScript 68.2%
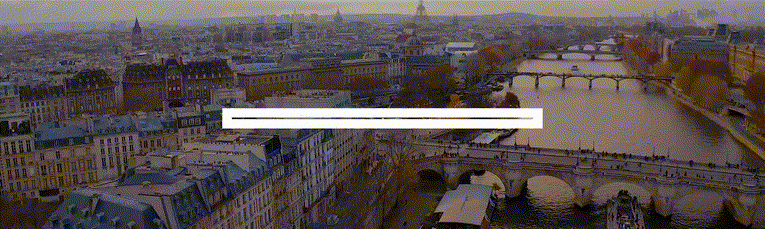
IMAGES
VIDEO
COMMENTS
We would like to show you a description here but the site won't allow us.
The Tour of Heroes tutorial takes you through the steps of creating an Angular application in TypeScript. The grand plan for this tutorial is to build an app that helps a staffing agency manage its stable of heroes. The Tour of Heroes app covers the core fundamentals of Angular. You'll build a basic app that has many of the features you'd ...
This Tour of Heroes tutorial shows you how to set up your local development environment and develop an app using the Angular CLI tool, and provides an introduction to the fundamentals of Angular.. The Tour of Heroes app that you build helps a staffing agency manage its stable of heroes. The app has many of the features you'd expect to find in any data-driven application.
CSS 20.9%. HTML 9.2%. Angular's sample app Tour of Heroes in v16. Contribute to dyave/angular-toh-16 development by creating an account on GitHub.
This Tour of Heroes tutorial provides an introduction to the fundamentals of Angular and shows you how to:. Set up your local Angular development environment. Use the Angular CLI to develop an application.; The Tour of Heroes application that you build helps a staffing agency manage its stable of heroes. The application has many of the features that you'd expect to find in any data-driven ...
Tutorial: Tour of Heroes. The grand plan for this tutorial is to build an app that helps a staffing agency manage its stable of heroes. The Tour of Heroes app covers the core fundamentals of Angular. You'll build a basic app that has many of the features you'd expect to find in a full-blown, data-driven app: acquiring and displaying a list ...
This Tour of Heroes tutorial shows you how to set up your local development environment and develop an application using the Angular CLI tool, and provides an introduction to the fundamentals of Angular.. The Tour of Heroes application that you build helps a staffing agency manage its stable of heroes. The application has many of the features you'd expect to find in any data-driven application.
Angular Tour of Heroes The Tour of Heroes tutorial covers the fundamentals of Angular. In this tutorial you will build an app that helps a staffing agency manage its stable of heroes. This basic app has many of the features you'd expect to find in a data-driven application. It acquires and displays a list of heroes, edits a selected hero's ...
The Tour of Heroes tutorial takes us through the steps of creating an Angular application in TypeScript. This page is not yet available in JavaScript. We recommend reading it in TypeScript. Next Step The Hero Editor. Powered by Google ©2010-2017. Code licensed under an MIT-style License.
The Tour of Heroes tutorial provides an introduction to the fundamentals of Angular. Details at https://angular.io/tutorial. Explore this online Tour of Heroes (Angular Example) sandbox and experiment with it yourself using our interactive online playground. You can use it as a template to jumpstart your development with this pre-built solution.
Become a Patreon! Your continued support enables me to continue creating free content: https://www.patreon.com/PaulHallidayIntroduction to Angular: Tour of H...
Tour of Heroes. This project was created to help represent a fundamental app written with Angular. The heroes and villains theme is used throughout the app. by John Papa. Comparative apps can be found here with Svelte, React, and Vue.
export class AppComponent {. title = 'Tour of Heroes'; } Compiling application & starting dev server…. angular-tour-of-heroes-example.stackblitz.io. Console. Clear on reload. The Tour of Heroes examples that covers the fundamentals of Angular.
The Tour of Heroes application has new requirements: Add a Dashboard view Add the ability to navigate between the Heroes and Dashboard views When users click a hero name in either view, navigate to a detail view of the selected hero When users click a deep link in an email, open the detail view for a particular hero When you're done, users ...
This tutorial provides an extensive overview of the Angular router. In this tutorial, you will build upon a basic router configuration to explore features such as child routes, route parameters, lazy load NgModules, guard routes, and preloading data to improve the user experience. For a working example of the final version of the app, see the .
Angular 17 has breaking changes with the Tour of Heroes tutorial on the original Angular.io docs site. My goal is to recreate the tutorial but using Angular 17 and standalone components. - awoerz/A...
angular.json. package.json. Using StackBlitz with your team? Join our livestream on June 5 to learn about using StackBlitz to securely collaborate with your organization. Save Your Spot. makagbdnnxg.angular.stackblitz.io. Console. Clear on reload. Angular Example - Tour of Heroes: Part 5.
This Tour of Heroes tutorial shows you how to set up your local development environment and develop an app using the Angular CLI tool, and provides an introduction to the fundamentals of Angular.. The Tour of Heroes app that you build helps a staffing agency manage its stable of heroes. The app has many of the features you'd expect to find in any data-driven application.
1. In the Tour of Heroes Part 0, it says. Run the CLI command ng new and provide the name angular-tour-of-heroes, as shown here: ng new angular-tour-of-heroes. However that prompts me for. Would you like to add Angular routing?
The Tour of Heroes tutorial provides an introduction to the fundamentals of Angular. Details at https://angular.io/tutorial. Explore this online tour-of-heroes-angular-example sandbox and experiment with it yourself using our interactive online playground. You can use it as a template to jumpstart your development with this pre-built solution.
Run ng e2e to execute the end-to-end tests via a platform of your choice. To use this command, you need to first add a package that implements end-to-end testing capabilities.
Run ng e2e to execute the end-to-end tests via a platform of your choice. To use this command, you need to first add a package that implements end-to-end testing capabilities.