Contact Mentor
Projects + Tutorials on React JS & JavaScript
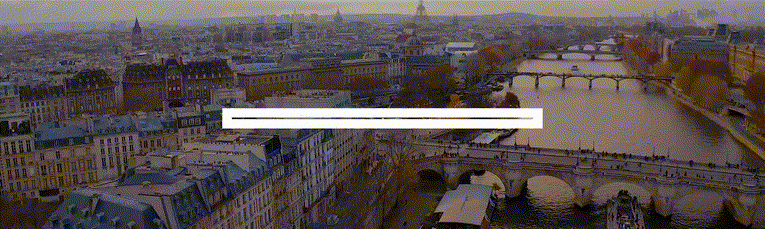
How to Build Website visitor counter in JavaScript with code
In this article, we will build a website visitor counter app with the following functionalities :
- Display the total number of visits on the page.
- Functionality to reset the number of visits on button click.
- Increment the count on refresh and future visits.
We will first start with creating HTML structure and then integrated it with JavaScript functionality which is followed by adding CSS styles.
1. Create <div> container to visitor count using HTML code
The title text is added to HTML file by adding wrapping it in <div></div>. In addition, we need to add another <div></div> with class “ website-counter ” with empty content for displaying visitor count using JavaScript.
2. DOM querySelector() to match the container using JavaScript
The next step is to use document.querySelector() to match the HTML element with the class “website-counter” and assign it to a variable for future DOM manipulation operations. Learn more about querySelector from developer.mozilla.org/Document/querySelector .
3. Retrieve visitor count using LocalStorage.getItem()
Now we retrieve the previous value of website visitor count from localStorage by using the localStorage.getItem() method by passing key “page_view” as a parameter. The value is stored in localStorage of the web browser and persists the data beyond a single session. Learn more about localStorage and its methods from tiny.cloud/blog/javascript-localstorage/ .
4. Initialize visitor count to 1 in localStorage if entry is not found
The localStorage entry is absent during first user session, hence localStorage.getItem(“page_view”) will return undefined . This case is handled by initializing the visitCount to 1 and adding the entry using localStorage.setItem(“”) with “page_view” as the key.
5. Increment visitor count and update the localStorage
On the contrary previous scenario, if an entry for the “page_view” key is already present , the retrieved String value is converted to a number datatype using Number(). The previous session visitCount is then incremented and the value is updated in localStorage.
6. Display visitor count on page using element.innerHTML
Once the correct value of visitCount is determined, it needs to be rendered on the screen . This is accomplished by assigning the visitCount variable to element.innerHTML . The .innerHTML property on selected DOM element allows HTML code to be added inside selected DOM element . As a result, the HTML code of the webpage will be updated to display the website visitor count. Learn more about element.innerHTML from developer.mozilla.org/Element/innerHTML .
7. Implement Reset functionality triggered by button click
Now that visitor count is implemented, we need to add a reset button to allow users to reset the counter back to 1 . The first step is to include the HTML code for the reset button and match the button using DOM querySelector() similar to “website-counter” .
The selected DOM element is attached with a click event listener to trigger the reset handler function. The website visitor count is reset by reinitializing visitCount to 1 and updating the value in localStorage. The visitor count is updated on the screen using element.innerHTML similar to previous step .
8. Add CSS Styles to website visitor counter
Once all the functionalities of the website visitor counter are implemented, it is now time to add CSS styles to the app in order to add a professional look and improve usability . The CSS file is then linked to the HTML webpage by using the <link> tag .
Refer Final code section below for the styles.css code.
Do you want to build an Amazing game in JavaScript? Read 6 Easy to build JavaScript Games for Beginners
Related Articles
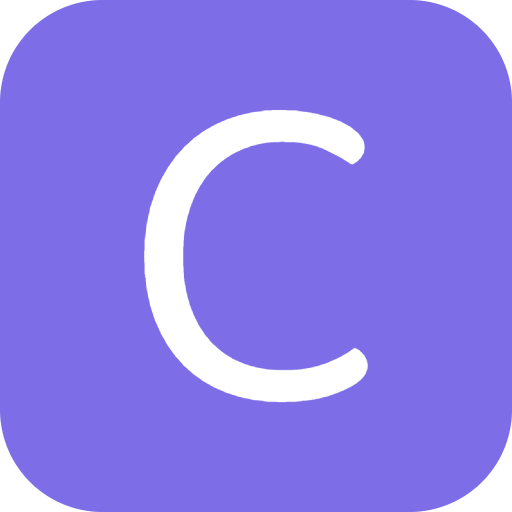

How to add or create a counter on your web page

Putting a hit counter (page counter) on a web page helps you track how many visitors visit your web page . There are several ways to go about putting a counter on your page, either by adding a pre-built one or creating your own.
We highly recommend using the Google Analytics over any web page counter, as it gives you more valuable information.
Adding a pre-built counter
If you prefer to add a pre-built counter to your web page, here are a few ways to do so.
- Check with the web host for your web page. Often the company hosting your web page offer free solutions and provide instructions on how to add the counter code to your web page.
- Acquire a pre-built counter from websites that offer free counters and add it to your web page with only a few lines of code. We recommend the following sites for free counters.
- Free counter stat
- Open a search for more free counter solutions.
Example of the free counter stat
A counter like the one used above increases in count if you or any visitor refreshes their browser. For example, if you refresh this page, the above counter would increase. If other people have visited since you first loaded the page, the counter may go up by several numbers.
Create a counter
To create a counter, you must create a Perl , PHP (PHP: Hypertext Preprocessor), or another script. Then, either refer to that script through a server-side include or another method. We recommend you already know or learn Perl or PHP programming to create the counter script.
The code for creating a counter can vary, depending on the programming language used and the type of counter you want to add to your web page. Below are some web pages that offer sample counter script code to use as a guide for creating a counter.
We have not tested the sample counter scripts provided on these web pages, so we cannot guarantee they're bug-free.
- Sample Perl counter script #1
- Sample PHP counter script #2
Related information
- How to get more traffic or people to visit my website.
- See the traffic and hit for further information about these terms and related links.
- HTML and web design help and support.
Creating a Counter with HTML, CSS, and JavaScript (Source Code)
By Faraz - October 10, 2023
Learn how to create an interactive counter for your website using HTML, CSS, and JavaScript. Perfect for web development beginners.
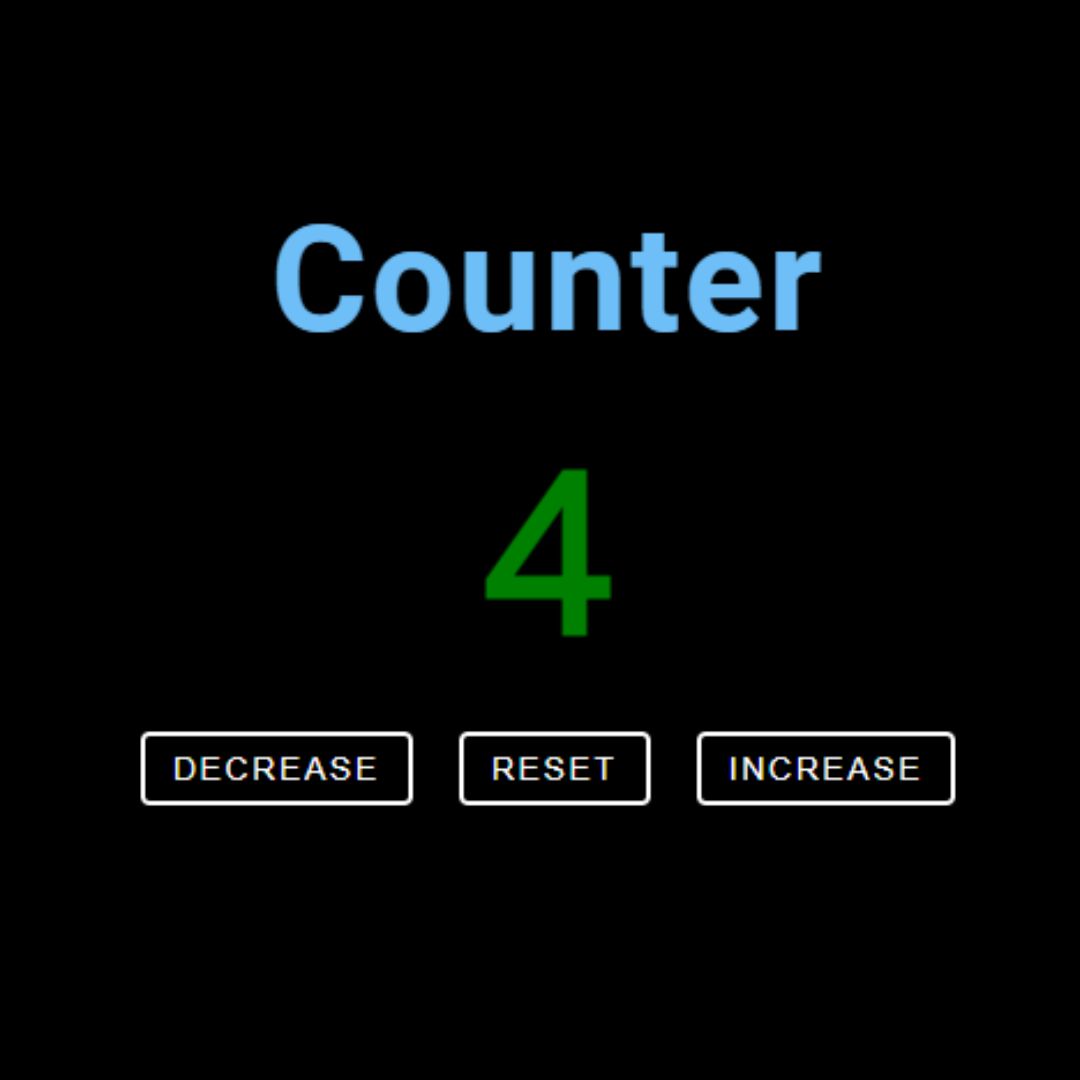
Table of Contents
- Project Introduction
- JavaScript Code
Are you a budding web developer eager to add interactive elements to your website? One great place to start is by creating a counter! In this tutorial, we will guide you through building a counter from scratch using HTML, CSS, and JavaScript. Whether you're a complete beginner or looking to expand your web development skills, this step-by-step guide is for you.
Let's start making a counter using HTML, CSS, and JavaScript step by step.
Join My Telegram Channel to Download the Project Source Code: Click Here
Prerequisites:
Before starting this tutorial, you should have a basic understanding of HTML, CSS, and JavaScript. Additionally, you will need a code editor such as Visual Studio Code or Sublime Text to write and save your code.
Source Code
Step 1 (HTML Code):
To get started, we will first need to create a basic HTML file. In this file, we will include the main structure for our counter.
After creating the files just paste the following codes into your file. Make sure to save your HTML document with a .html extension, so that it can be properly viewed in a web browser.
Let's break it down step by step:
1. <!DOCTYPE html> : This declaration specifies the document type and version of HTML being used. In this case, it's HTML5.
2. <html lang="en"> : This is the root element of the HTML document. The lang attribute specifies the language of the document, which is set to English (en).
3. <head> : This section contains metadata about the web page, which is not visible to users but is important for browsers and search engines.
- <meta charset="UTF-8"> : This meta tag defines the character encoding for the document, which is UTF-8. UTF-8 is a character encoding that supports a wide range of characters, including those from various languages.
- <meta name="viewport" content="width=device-width, initial-scale=1.0"> : This meta tag sets the viewport settings for responsive web design. It tells the browser to adjust the width of the page to the device's screen width and sets the initial zoom level to 1.0.
- <title> Counter </title> : This sets the title of the web page to "Counter," which appears in the browser's title bar or tab.
- <link rel="stylesheet" href="styles.css"> : This line includes an external stylesheet called "styles.css." Stylesheets are used to define the visual presentation (e.g., colors, fonts) of the web page.
4. <body id="body"> : This is the body of the web page where the visible content is placed.
5. <button type="button" class="btn changemode"> Switch Dark mode </button> : This is a button element with a class "btn" and "changemode." It will be used to toggle between dark and light modes on the page.
6. <main> : This is a semantic HTML5 element that represents the main content of the web page.
7. <div class="container"> : This is a div element with a class "container" that serves as a container for the content within it.
8. <h1> counter </h1> : This is a level 1 heading element that displays the text "counter."
9. <span id="value"> 0 </span> : This is a span element with an id "value" that initially displays the number "0." It can be used to dynamically update and display a counter value.
10. <div class="button-container"> : This div with the class "button-container" serves as a container for a group of buttons.
11. Three buttons:
- <button class="btn decrease"> decrease </button> : A button with a class "btn" and "decrease" that is used to decrease the counter value.
- <button class="btn reset"> reset </button> : A button with a class "btn" and "reset" that is used to reset the counter value.
- <button class="btn increase"> increase </button> : A button with a class "btn" and "increase" that is used to increase the counter value.
12. <script src="script.js"> </script> : This line includes an external JavaScript file called "script.js." JavaScript is used to add interactivity and functionality to the web page.
This is the basic structure of our counter using HTML, and now we can move on to styling it using CSS.
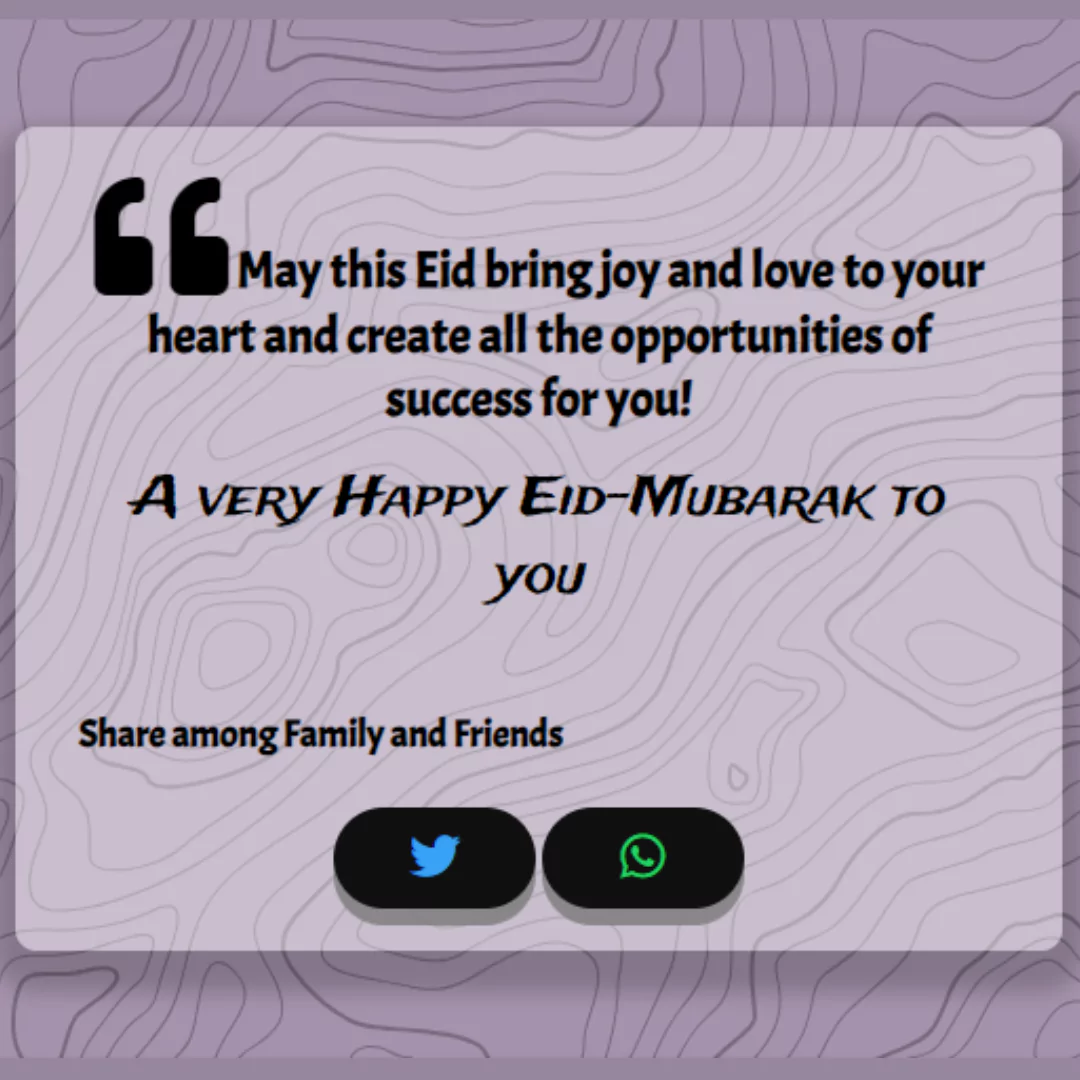
Step 2 (CSS Code):
Once the basic HTML structure of the counter is in place, the next step is to add styling to the counter using CSS.
Next, we will create our CSS file. In this file, we will use some basic CSS rules to style our counter. Let's break down what each part of the code does:
1. @import url("https://fonts.googleapis.com/css?family=Open+Sans|Roboto:400,700&display=swap");
- This line imports two Google Fonts, "Open Sans" and "Roboto" with two weights (400 and 700) for later use in the CSS.
2. :root { ... }
- This defines CSS custom properties (variables) inside the :root pseudo-class. These variables are used to store values that can be reused throughout the stylesheet.
3. *, ::after, ::before { ... }
- These selectors are used to reset the default margin, padding, and box-sizing for all elements on the page, ensuring a consistent layout.
4. body { ... }
- Defines styles for the body element, including font family, background color, text color, line-height, and font size.
5. ul { ... }
- Styles for unordered lists to remove bullets.
6. a { ... }
- Styles for anchor (link) elements to remove underlines.
7. h1, h2, h3, h4 { ... }
- Styles for headings (h1, h2, h3, h4) including letter spacing, text transformation, line height, margin, and font family. These styles make headings look consistent and visually appealing.
8. Media Queries (@media screen and (min-width: 800px) { ... })
- These styles apply only when the screen width is at least 800px. It adjusts font sizes and line heights for headings and body text to make the design responsive.
9. .section { ... }
- Styles for elements with the class "section," including padding.
10. .section-center { ... }
- Styles for elements with the class "section-center," setting the width and margins to center the content. It also limits the maximum width to 1170px.
11. .container { ... }
- Styles for elements with the class "container," defining text alignment.
12. #value { ... }
- Styles for an element with the ID "value," setting the font size and font weight.
13. .btn { ... }
- Styles for elements with the class "btn" (buttons), including text transformation, background color, text color, padding, letter spacing, and various other properties for button styling.
14. .btn:hover { ... }
- Styles for button hover states, changing the text and background colors to create an interactive effect when a user hovers over a button.
15. .darkbody { ... } and .darkbutton { ... }
- These classes define styles for creating a dark mode theme. They change the background color, text color, and border color for specific elements to create a dark color scheme.
16. .darkbutton:hover { ... }
- Styles for the dark mode button hover state, changing text and background colors when hovering over a button in dark mode.
This will give our counter an upgraded presentation. Create a CSS file with the name of styles.css and paste the given codes into your CSS file. Remember that you must create a file with the .css extension.
Step 3 (JavaScript Code):
Finally, we need to create a function in JavaScript. Let's break down the code step by step:
1. The code starts by selecting elements from the HTML document using the document.getElementById and document.querySelector methods:
- const value: This variable represents an HTML element with the id 'value', presumably a text element that displays a numeric value.
- const btn1, const btn2, const btn3: These variables represent buttons with class names 'decrease', 'reset', and 'increase', respectively. These buttons are used to decrease, reset, and increase the displayed value.
- const btnmode: This variable represents a button with the class name ' changemode '. This button is used to toggle between light and dark modes.
2. A variable number is declared and initialized to 0. This variable will store the numeric value that is displayed on the web page.
3. Event listeners are added to the buttons to handle user interactions:
- btn1.addEventListener('click', function() { ... }): When the 'decrease' button is clicked, this function is executed. It decreases the number variable by 1, updates the text content of the 'value' element, and changes the text color to red.
- btn2.addEventListener('click', function() { ... }): When the 'reset' button is clicked, this function is executed. It sets the number variable and the text content of the 'value' element to 0 and changes the text color to a shade of blue.
- btn3.addEventListener('click', function() { ... }): When the 'increase' button is clicked, this function is executed. It increases the number variable by 1, updates the text content of the 'value' element, and changes the text color to green.
4. Another event listener is added to the btnmode button:
- btnmode.addEventListener('click', function() { ... }): When the 'changemode' button is clicked, this function is executed. It toggles a CSS class 'darkbody' on the 'body' element, effectively switching between light and dark modes. It also changes the text content of the 'btnmode' button to toggle between "Switch Light Mode" and "Switch Dark Mode". Additionally, it toggles a 'darkbutton' class on the buttons (btn1, btn2, btn3, and btnmode) to adjust their appearance in dark mode.
Create a JavaScript file with the name script.js and paste the given codes into your JavaScript file and make sure it's linked properly to your HTML document so that the scripts are executed on the page. Remember, you’ve to create a file with .js extension.
Final Output:
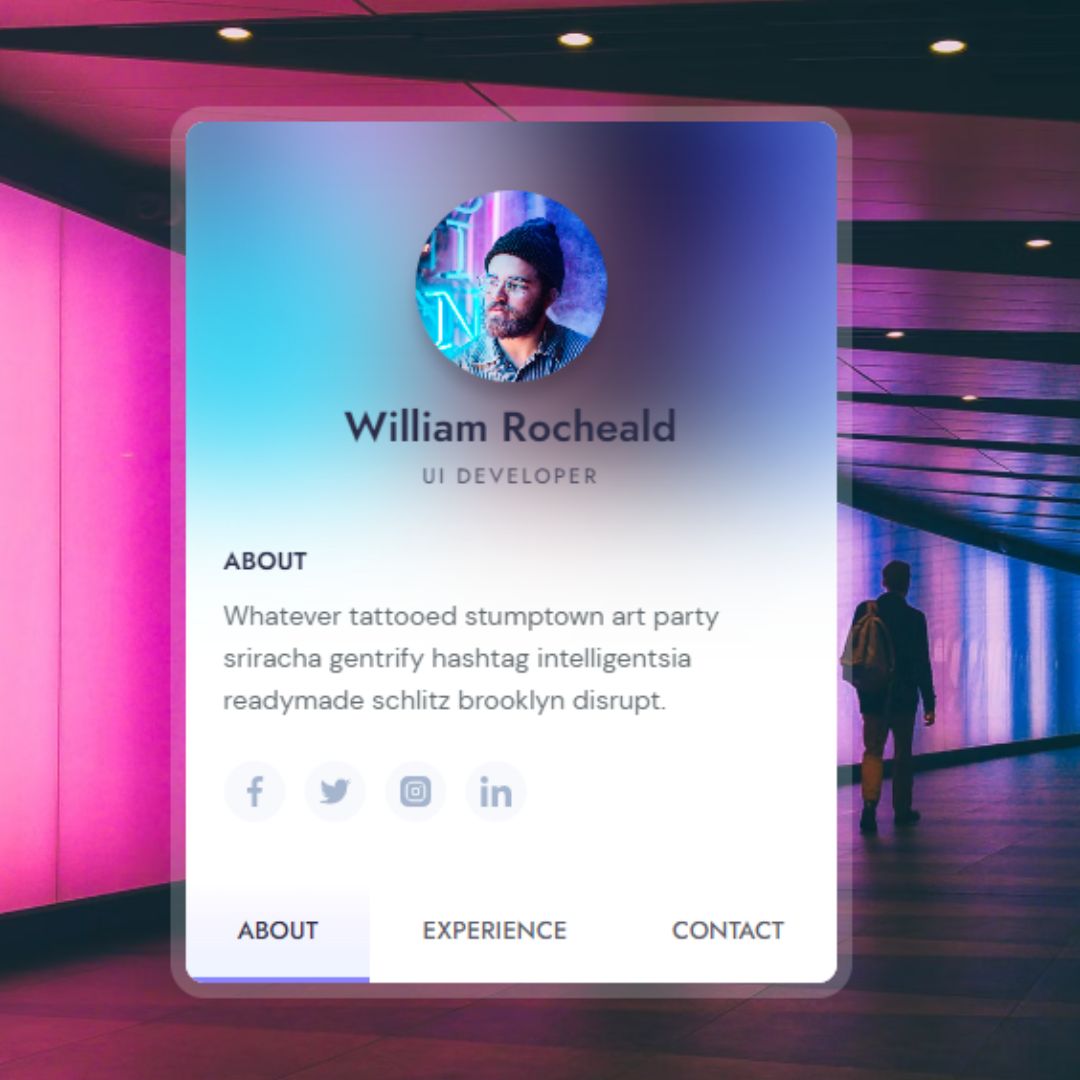
Conclusion:
Congratulations! You've successfully created a counter using HTML, CSS, and JavaScript. This tutorial is just the beginning of your web development journey. Feel free to experiment, customize, and add more features to your counter. Happy coding!
After completing this tutorial, you're on your way to becoming a skilled web developer. Stay curious and keep building!
That’s a wrap!
I hope you enjoyed this post. Now, with these examples, you can create your own amazing page.
Did you like it? Let me know in the comments below 🔥 and you can support me by buying me a coffee.
And don’t forget to sign up to our email newsletter so you can get useful content like this sent right to your inbox!
Thanks! Faraz 😊
Subscribe to my Newsletter
Get the latest posts delivered right to your inbox, latest post.
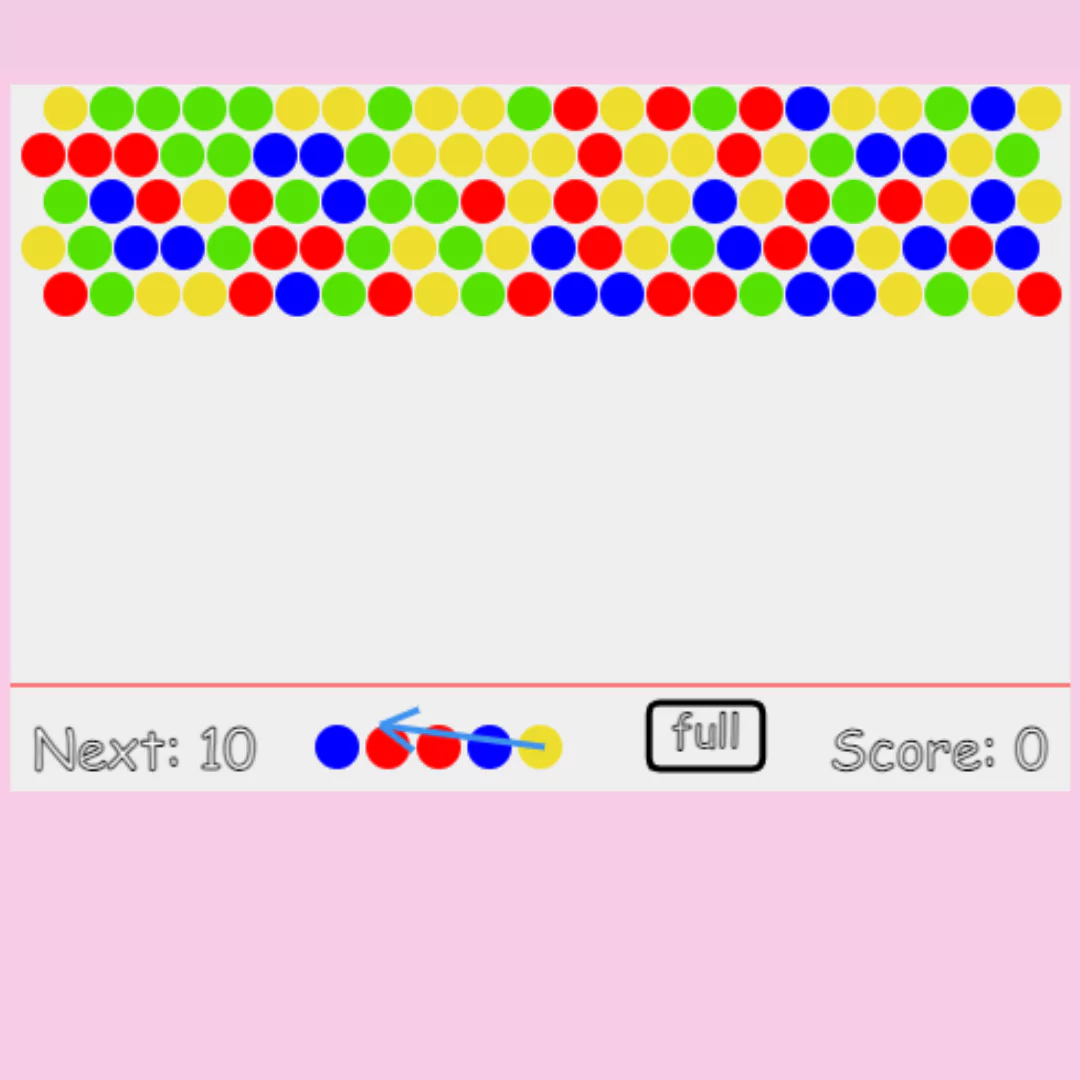
Create Your Own Bubble Shooter Game with HTML and JavaScript
Learn how to develop a bubble shooter game using HTML and JavaScript with our easy-to-follow tutorial. Perfect for beginners in game development.
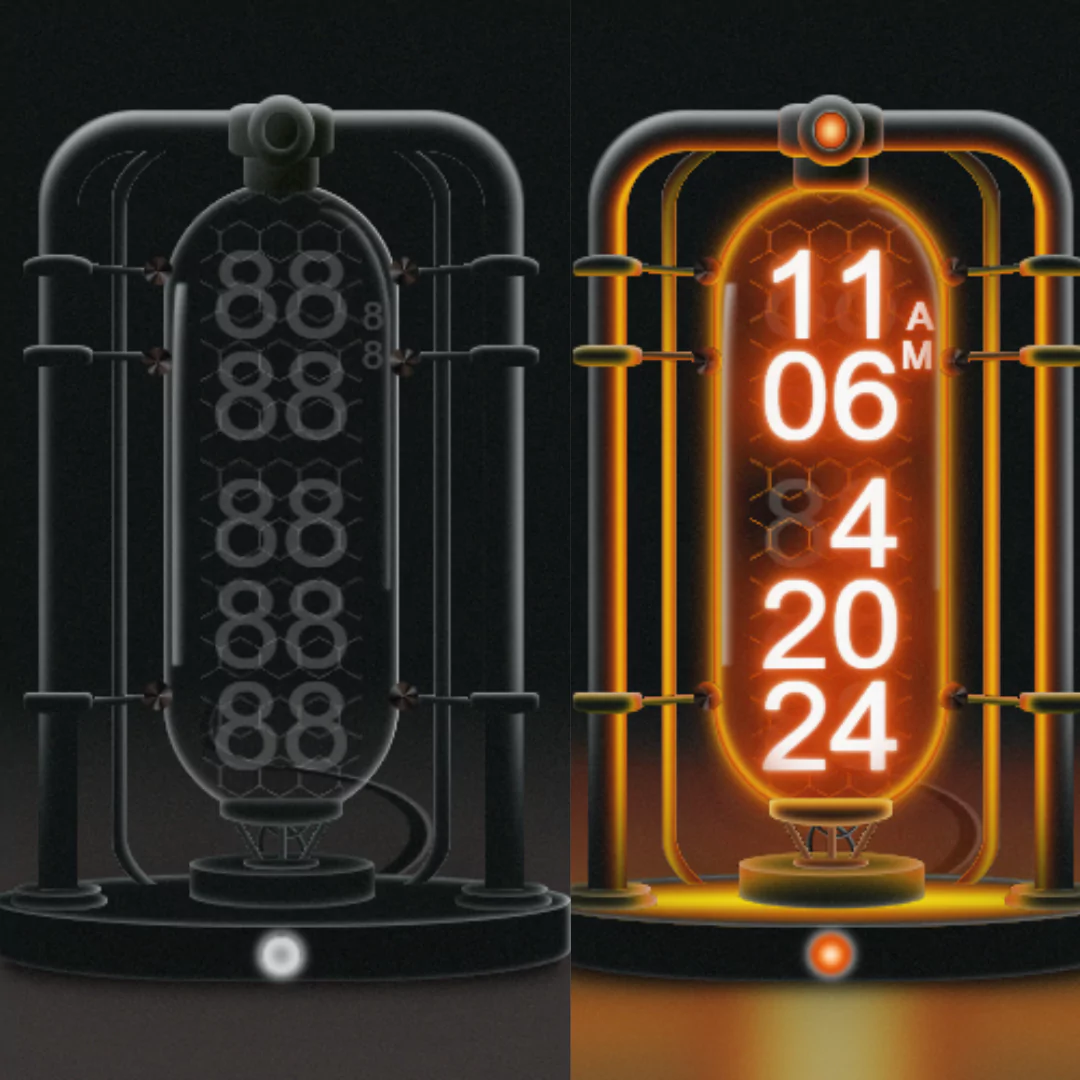
Build Your Own Nixie Tube Clock using HTML, CSS, and JavaScript (Source Code)
April 20, 2024
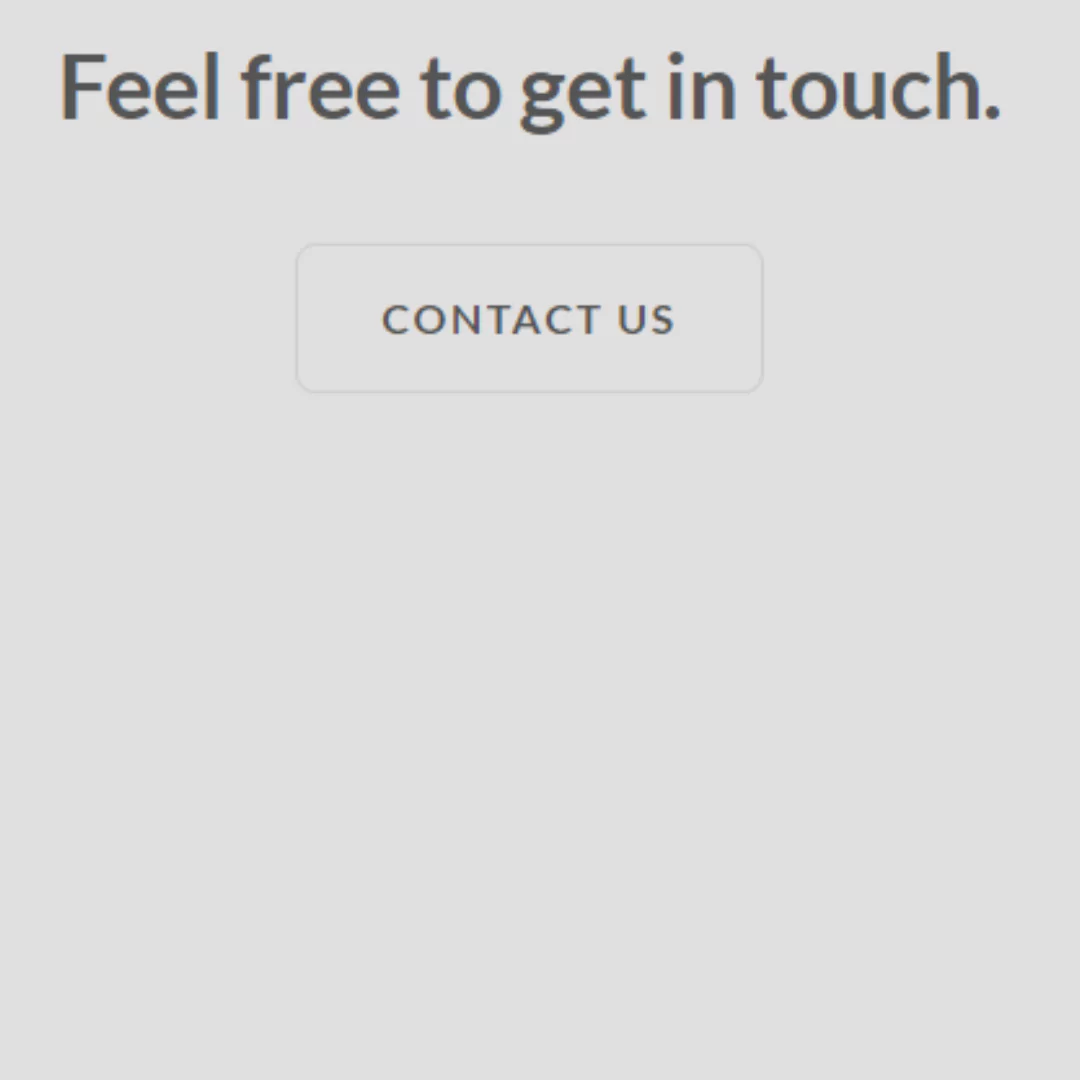
Create a Responsive Popup Contact Form: HTML, CSS, JavaScript Tutorial
April 17, 2024
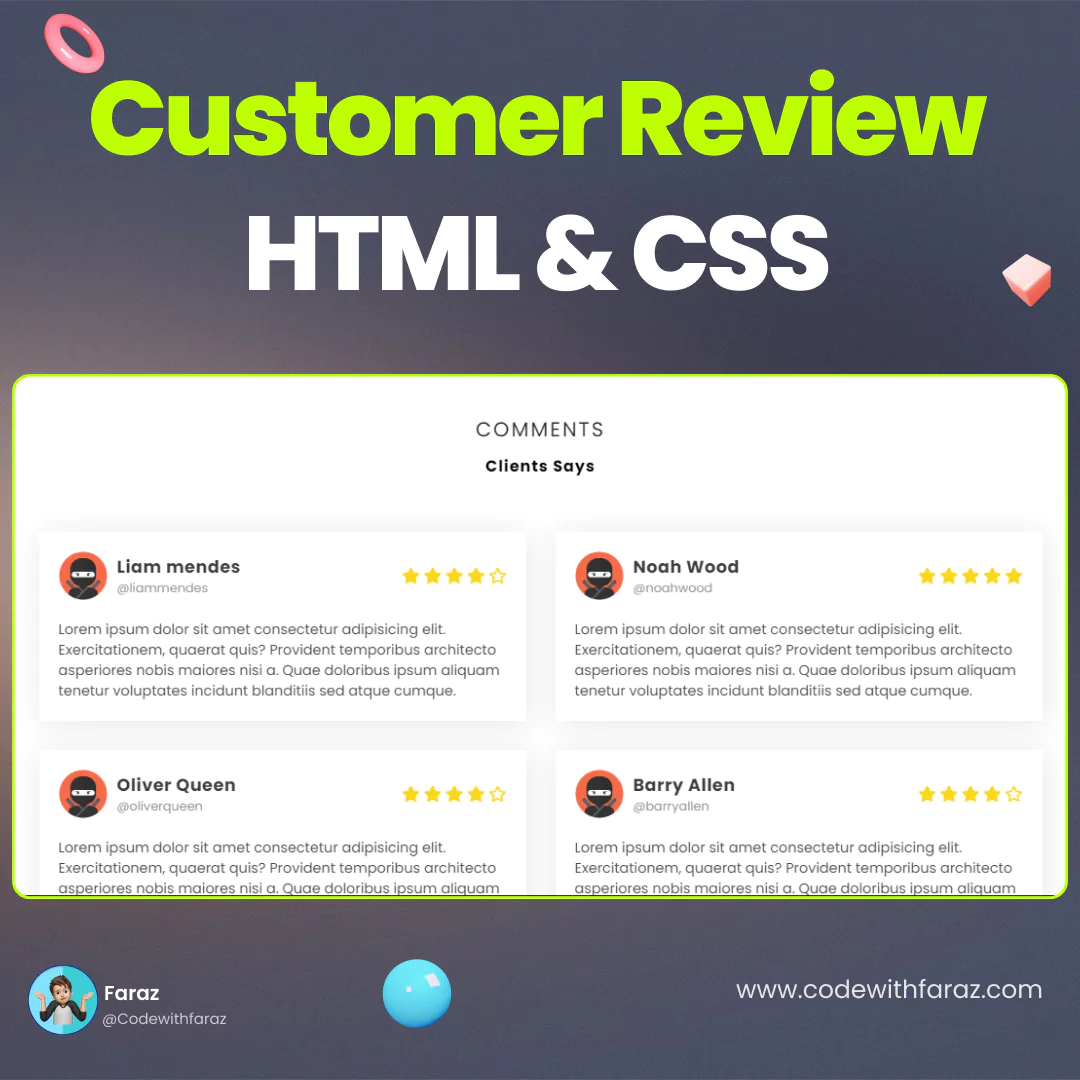
Create a Responsive Customer Review Using HTML and CSS
April 14, 2024
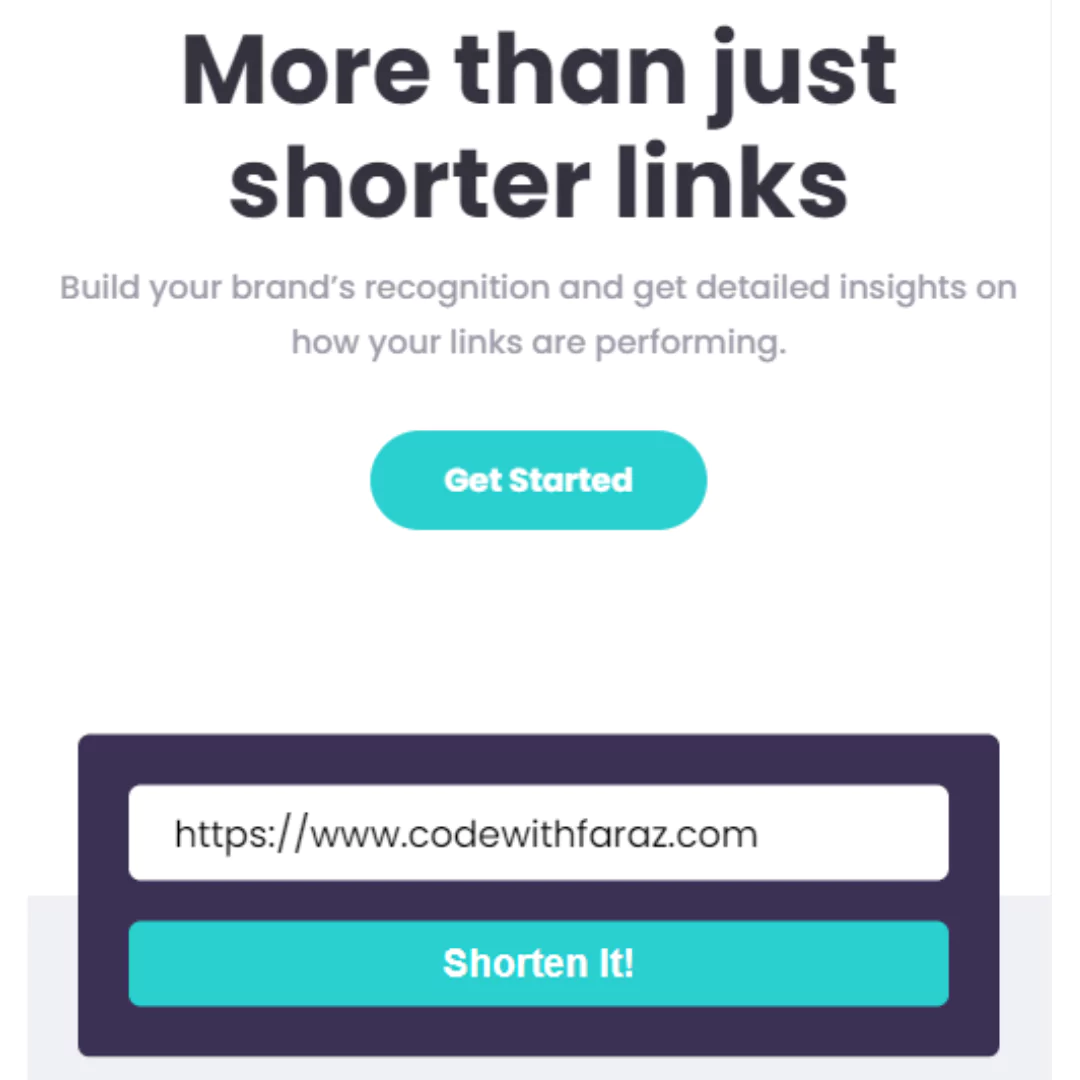
Create a URL Shortening Landing Page using HTML, CSS, and JavaScript
April 08, 2024
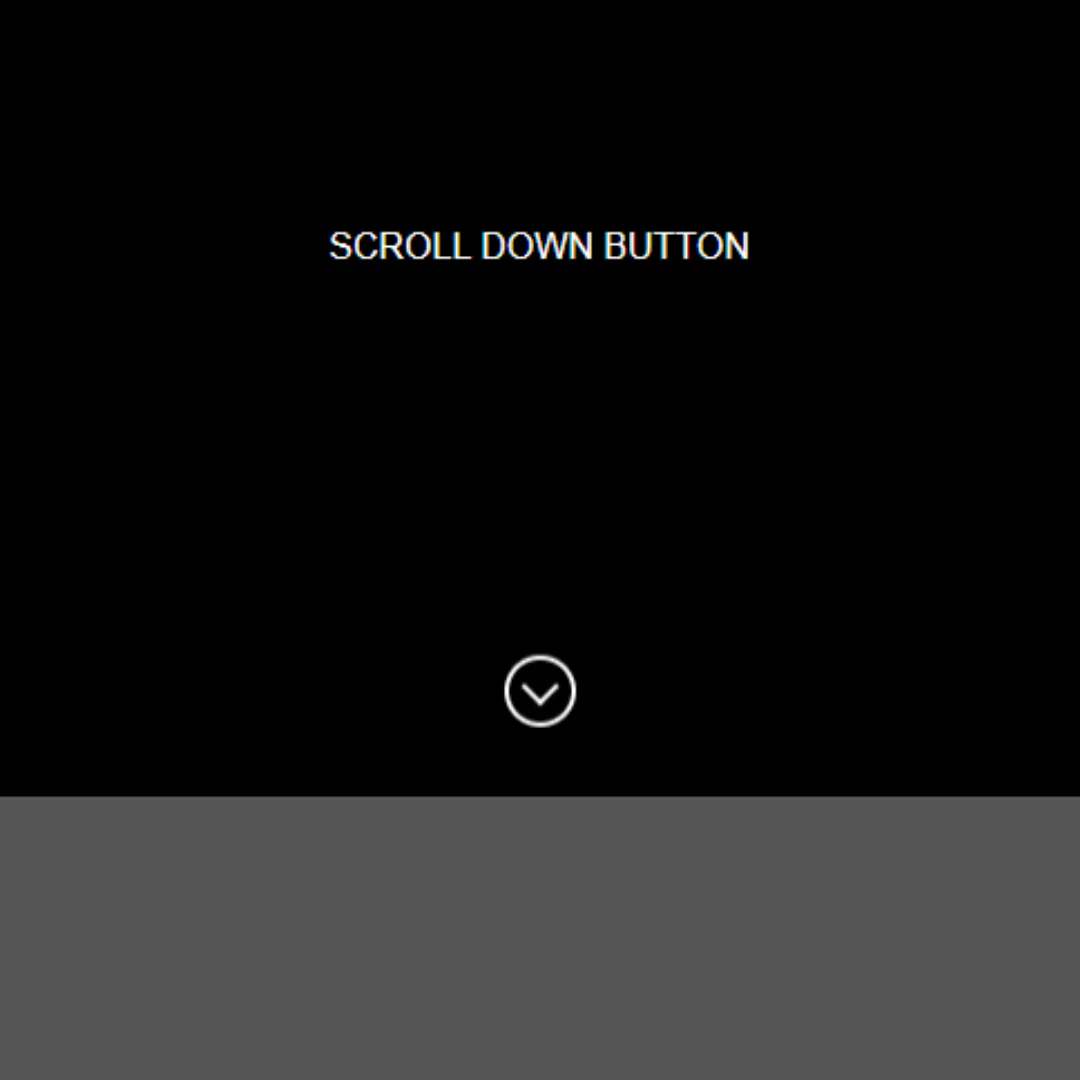
How to Create a Scroll Down Button: HTML, CSS, JavaScript Tutorial
Learn to add a sleek scroll down button to your website using HTML, CSS, and JavaScript. Step-by-step guide with code examples.
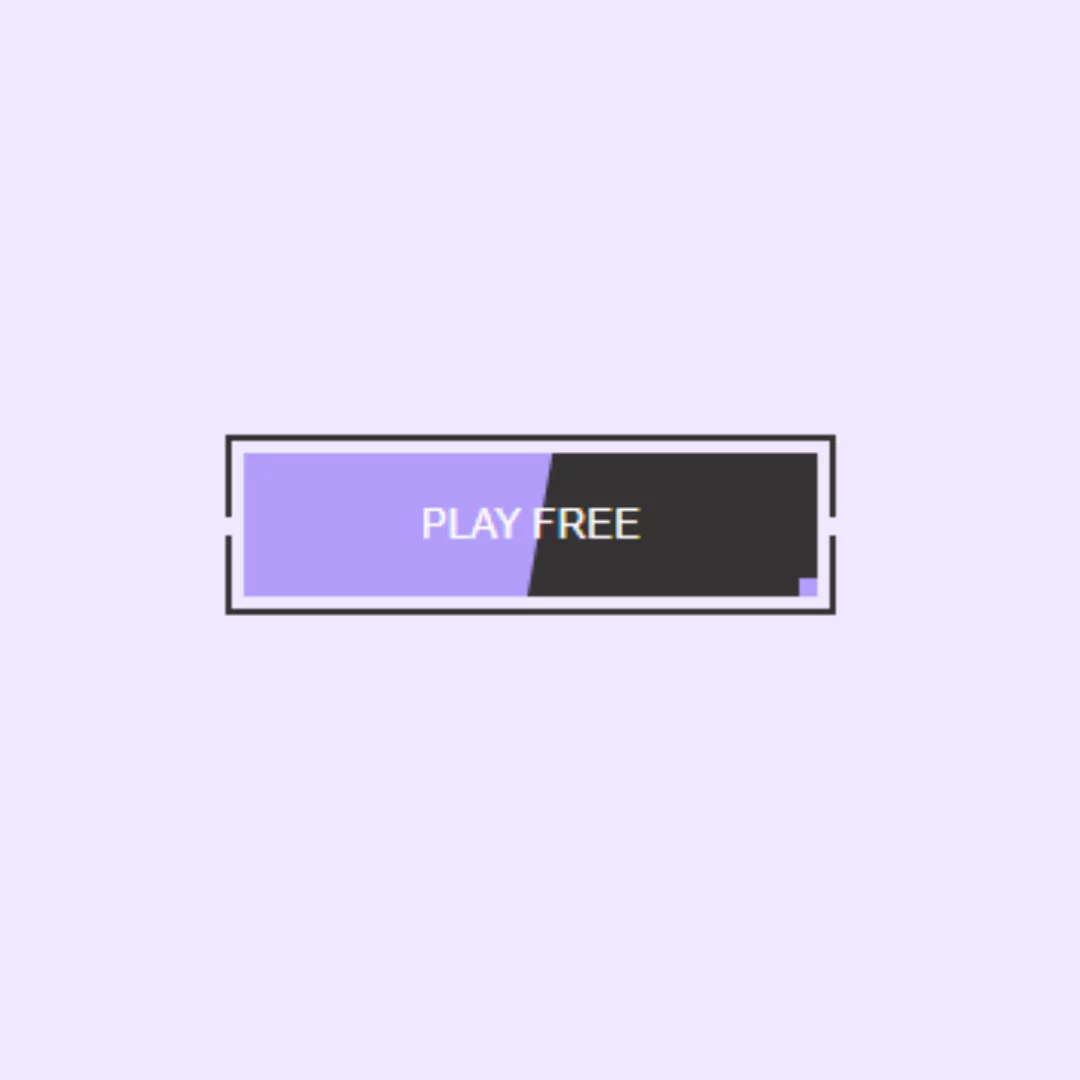
How to Create a Trending Animated Button Using HTML and CSS
March 15, 2024
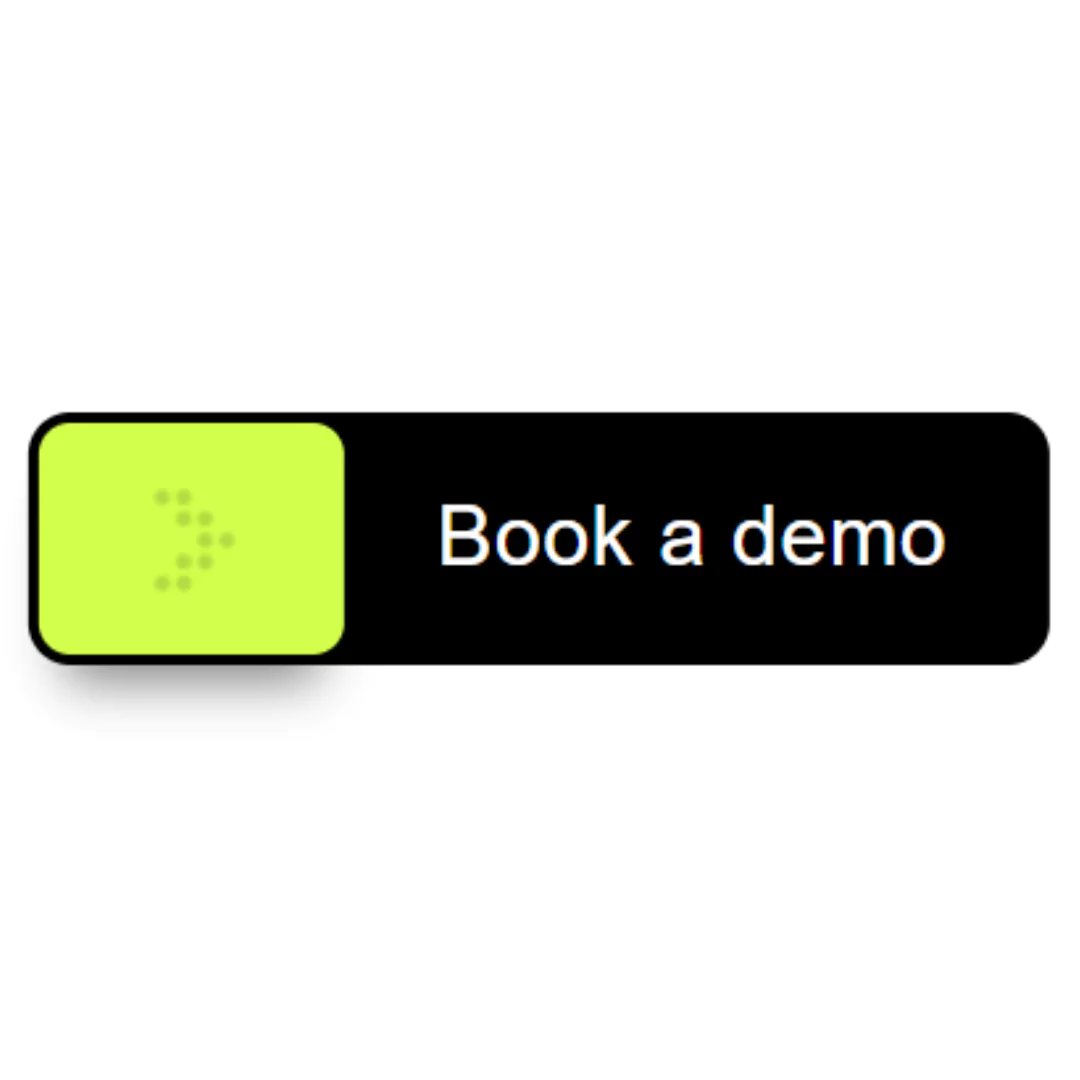
Create Interactive Booking Button with mask-image using HTML and CSS (Source Code)
March 10, 2024
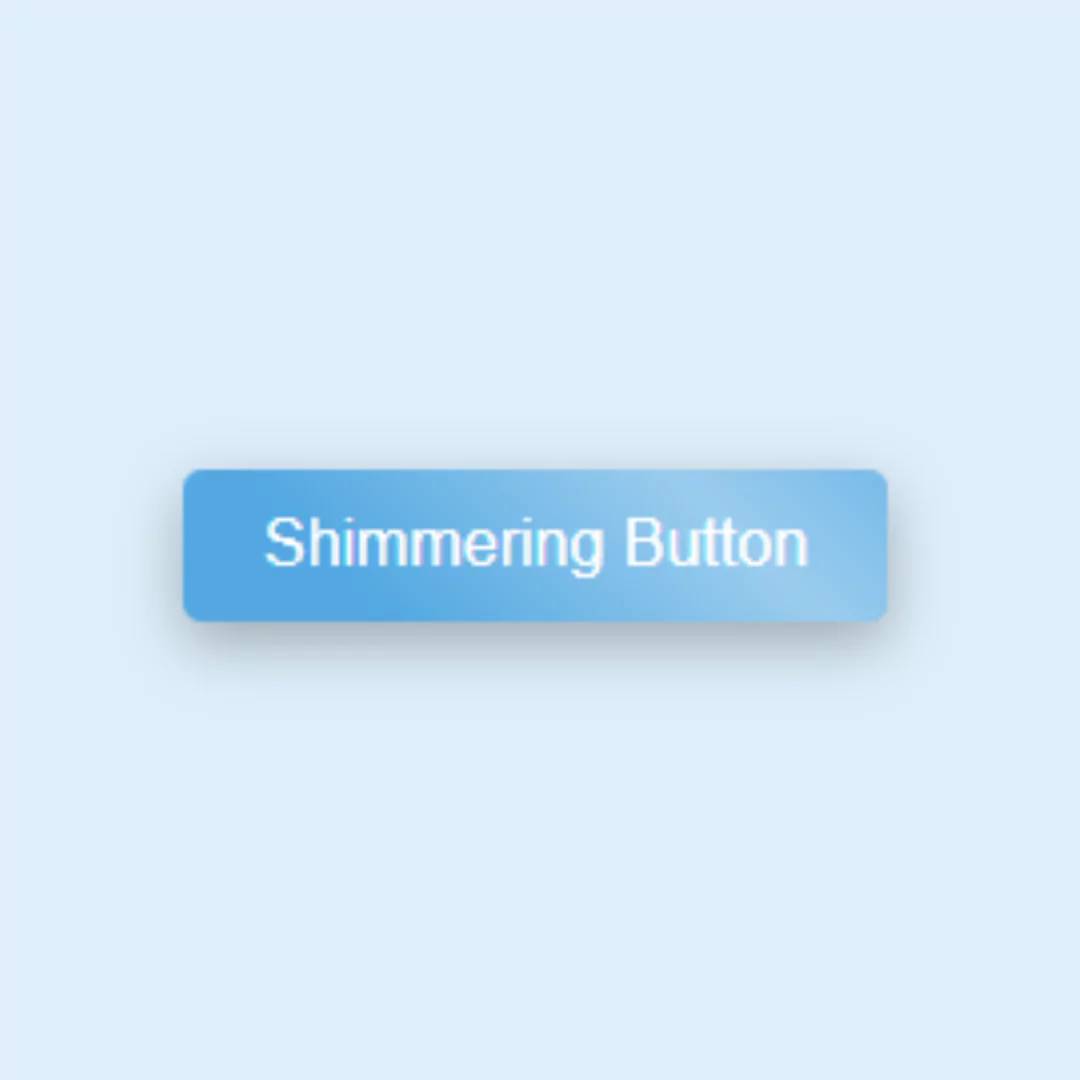
Create Shimmering Effect Button: HTML & CSS Tutorial (Source Code)
March 07, 2024
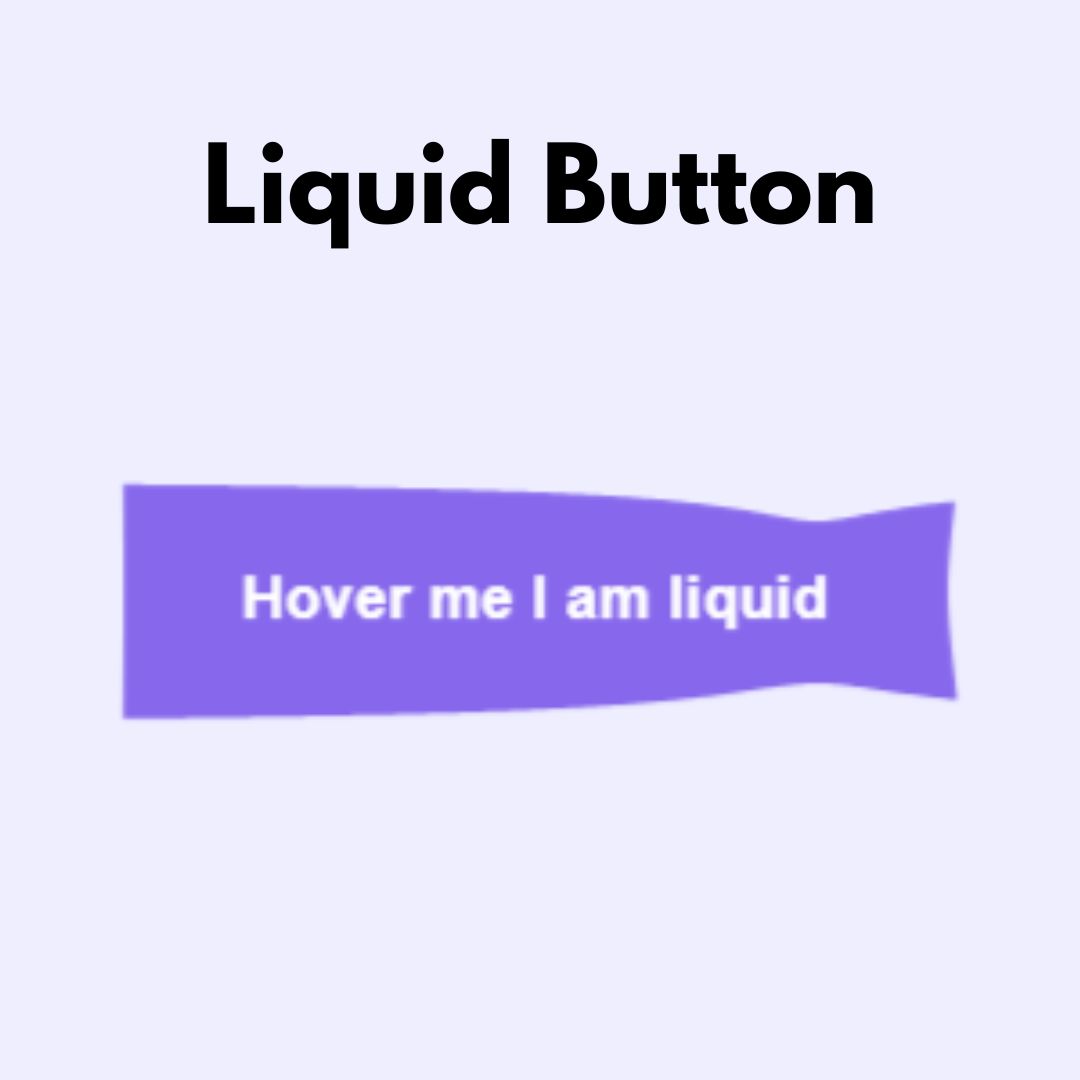
How to Create a Liquid Button with HTML, CSS, and JavaScript (Source Code)
March 01, 2024
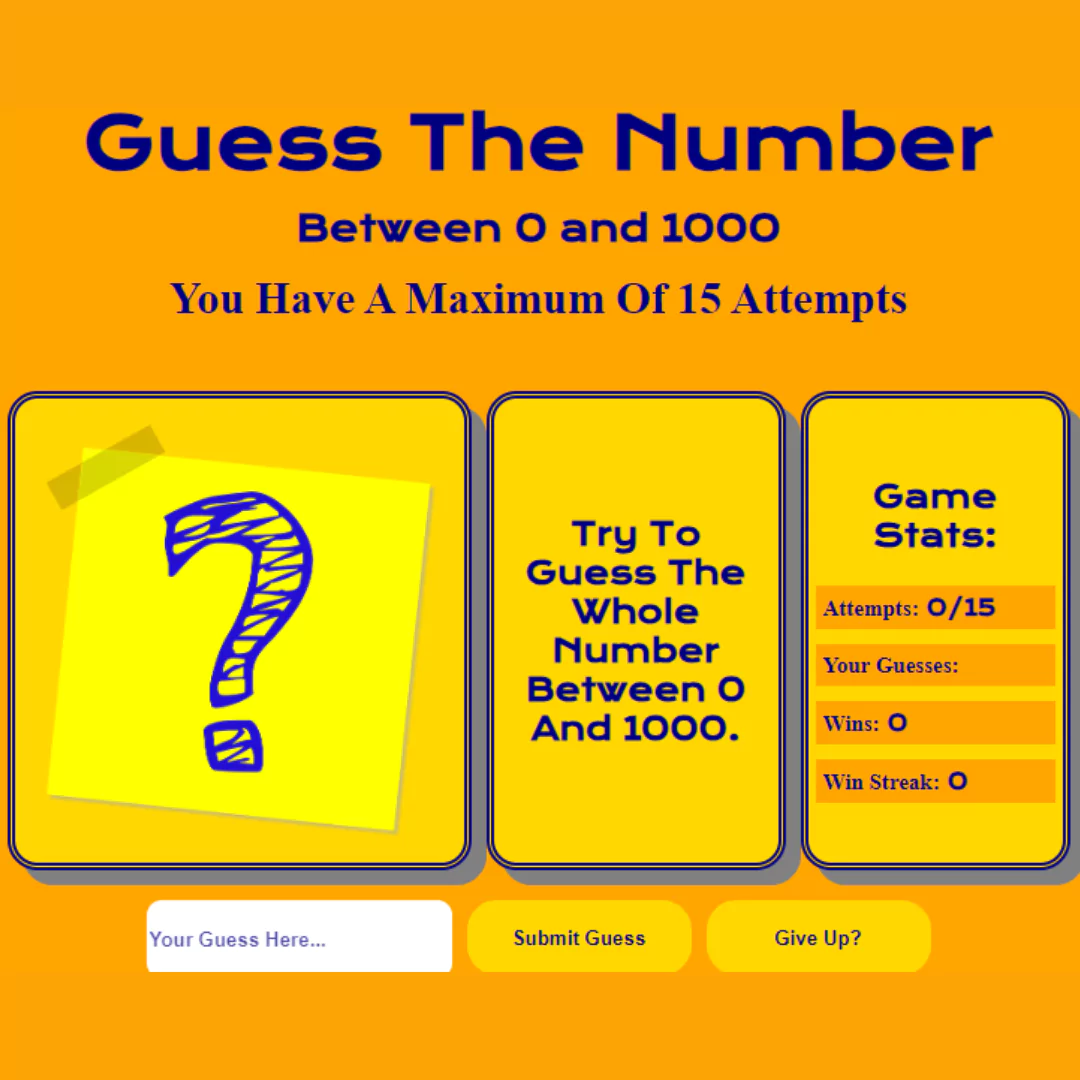
Build a Number Guessing Game using HTML, CSS, and JavaScript | Source Code
April 01, 2024
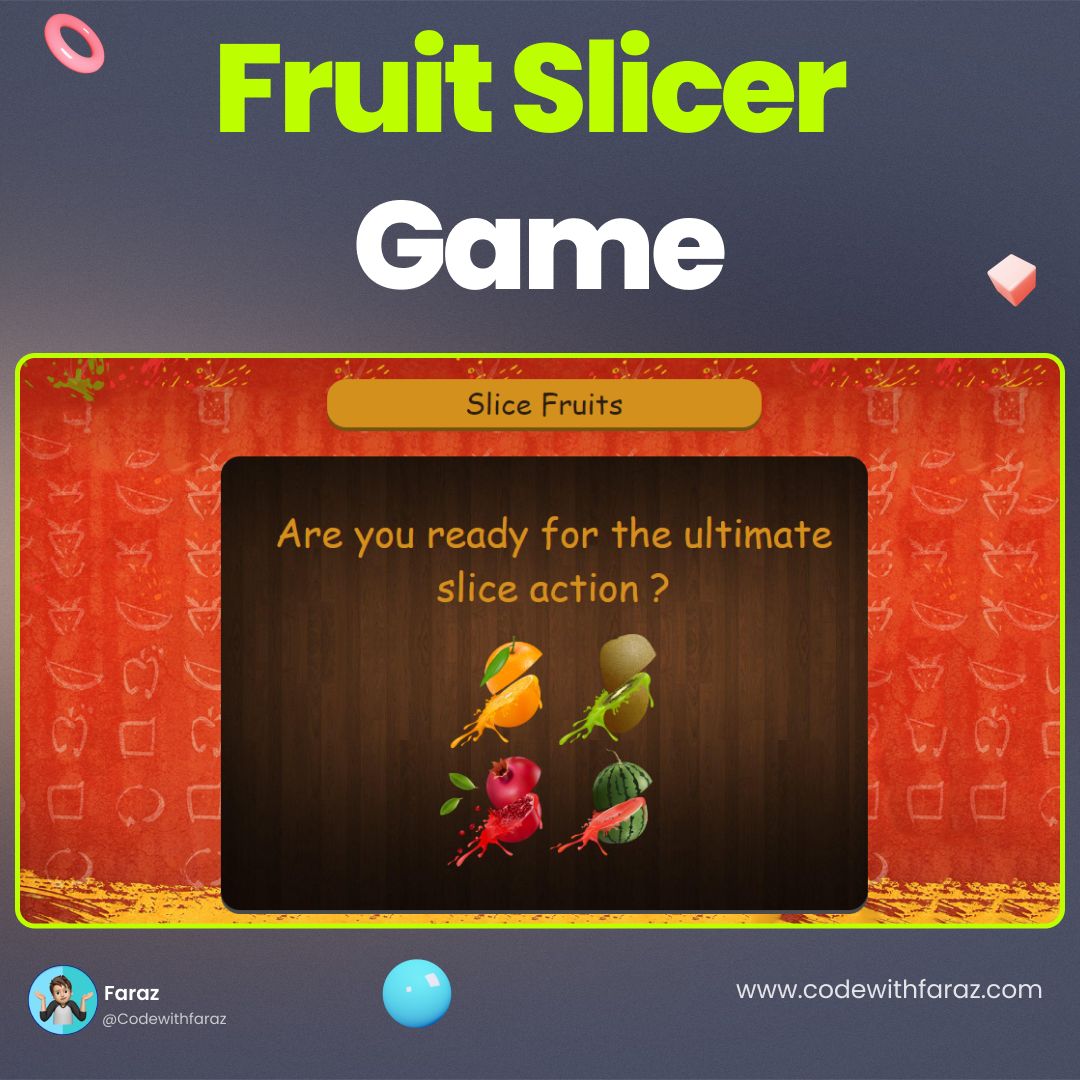
Building a Fruit Slicer Game with HTML, CSS, and JavaScript (Source Code)
December 25, 2023
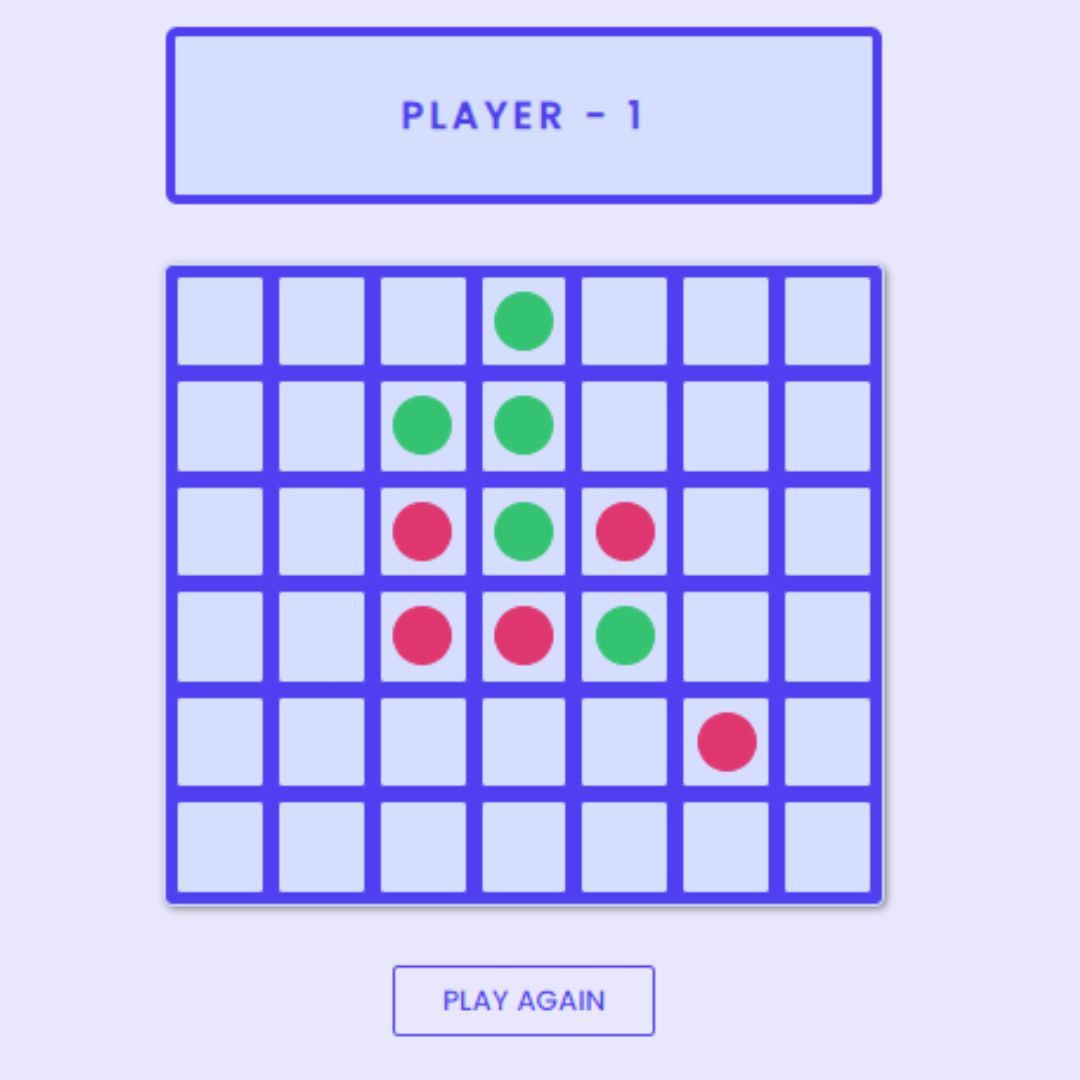
Create Connect Four Game Using HTML, CSS, and JavaScript (Source Code)
December 07, 2023
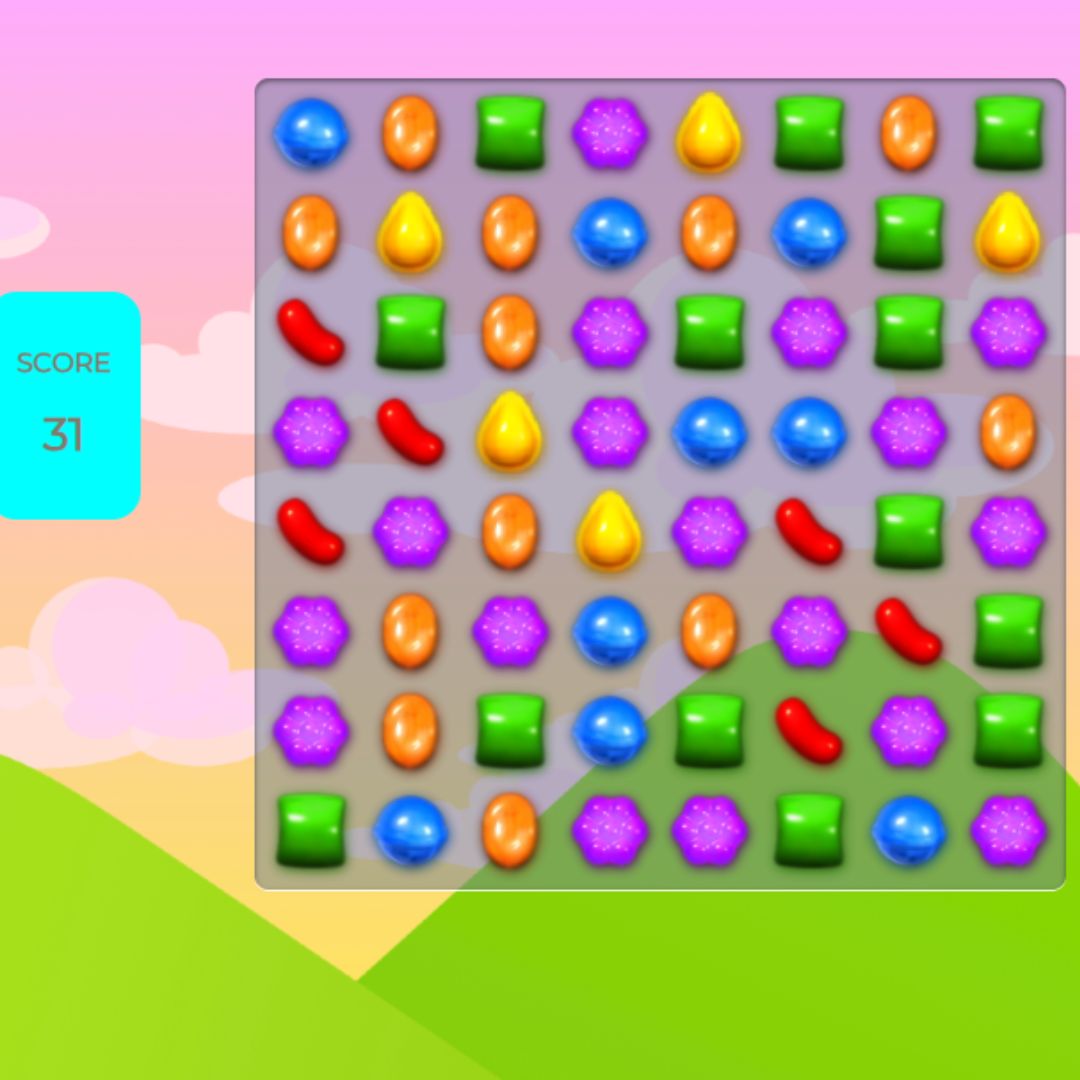
Creating a Candy Crush Clone: HTML, CSS, and JavaScript Tutorial (Source Code)
November 17, 2023
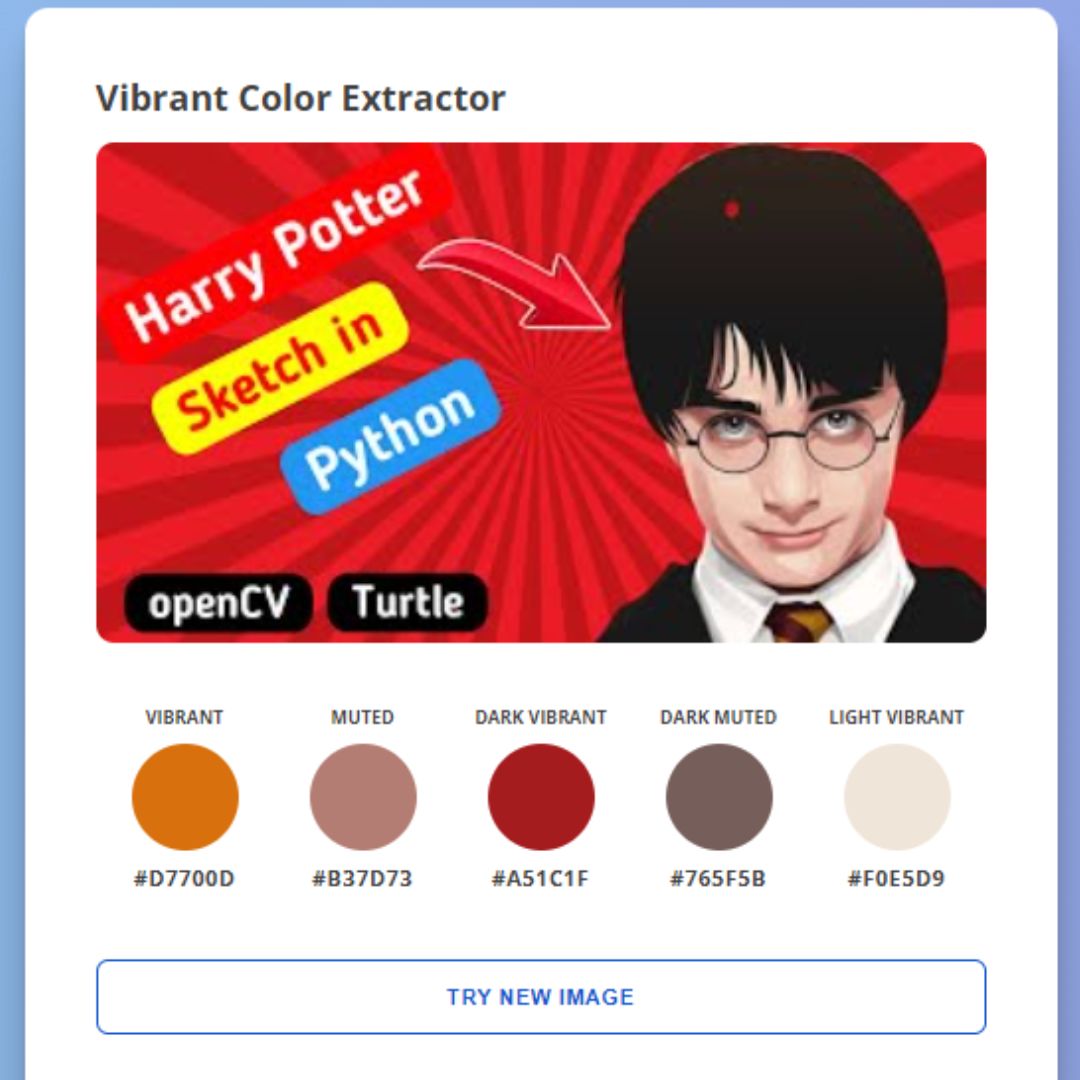
Create Image Color Extractor Tool using HTML, CSS, JavaScript, and Vibrant.js
Master the art of color picking with Vibrant.js. This tutorial guides you through building a custom color extractor tool using HTML, CSS, and JavaScript.
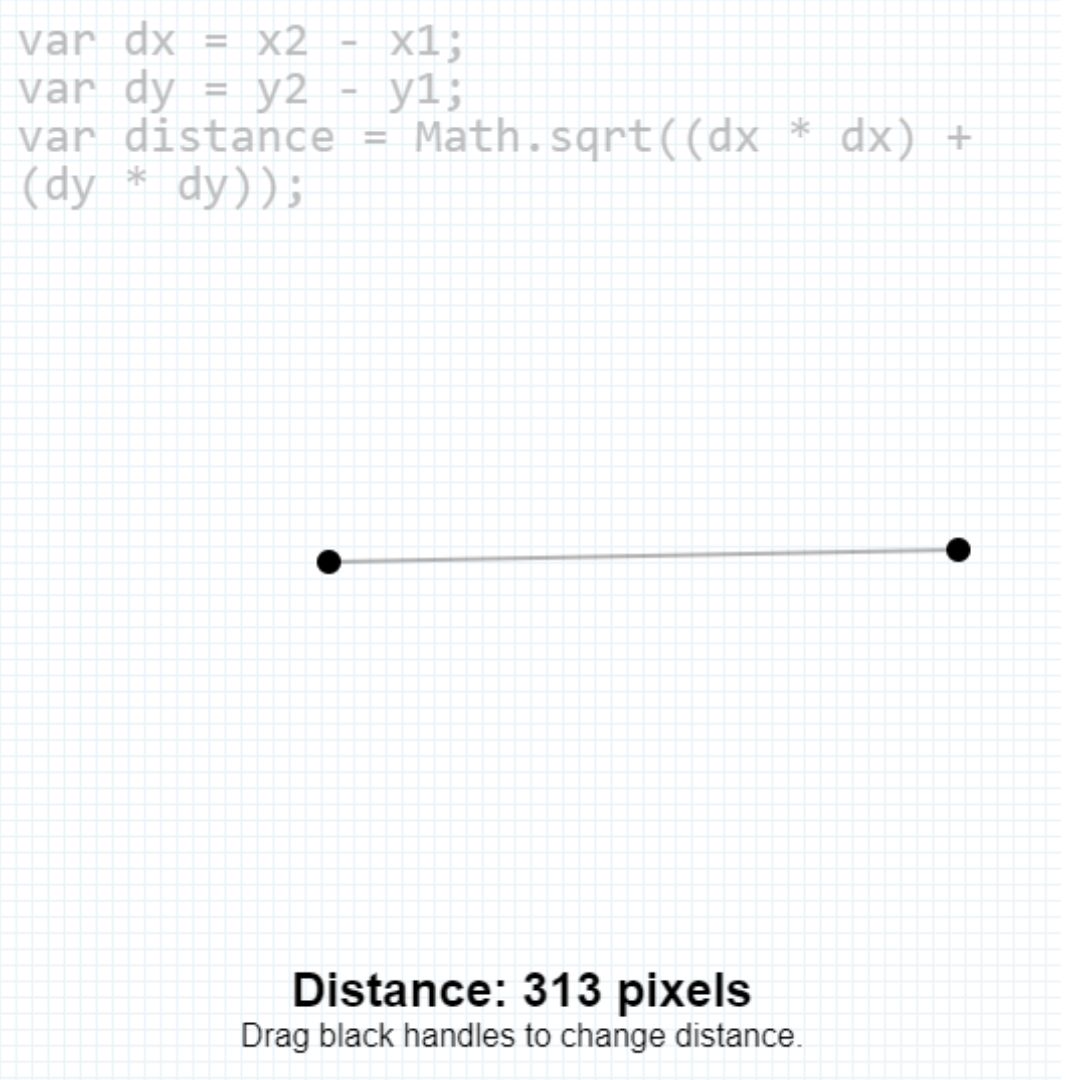
Build a Responsive Screen Distance Measure with HTML, CSS, and JavaScript
January 04, 2024
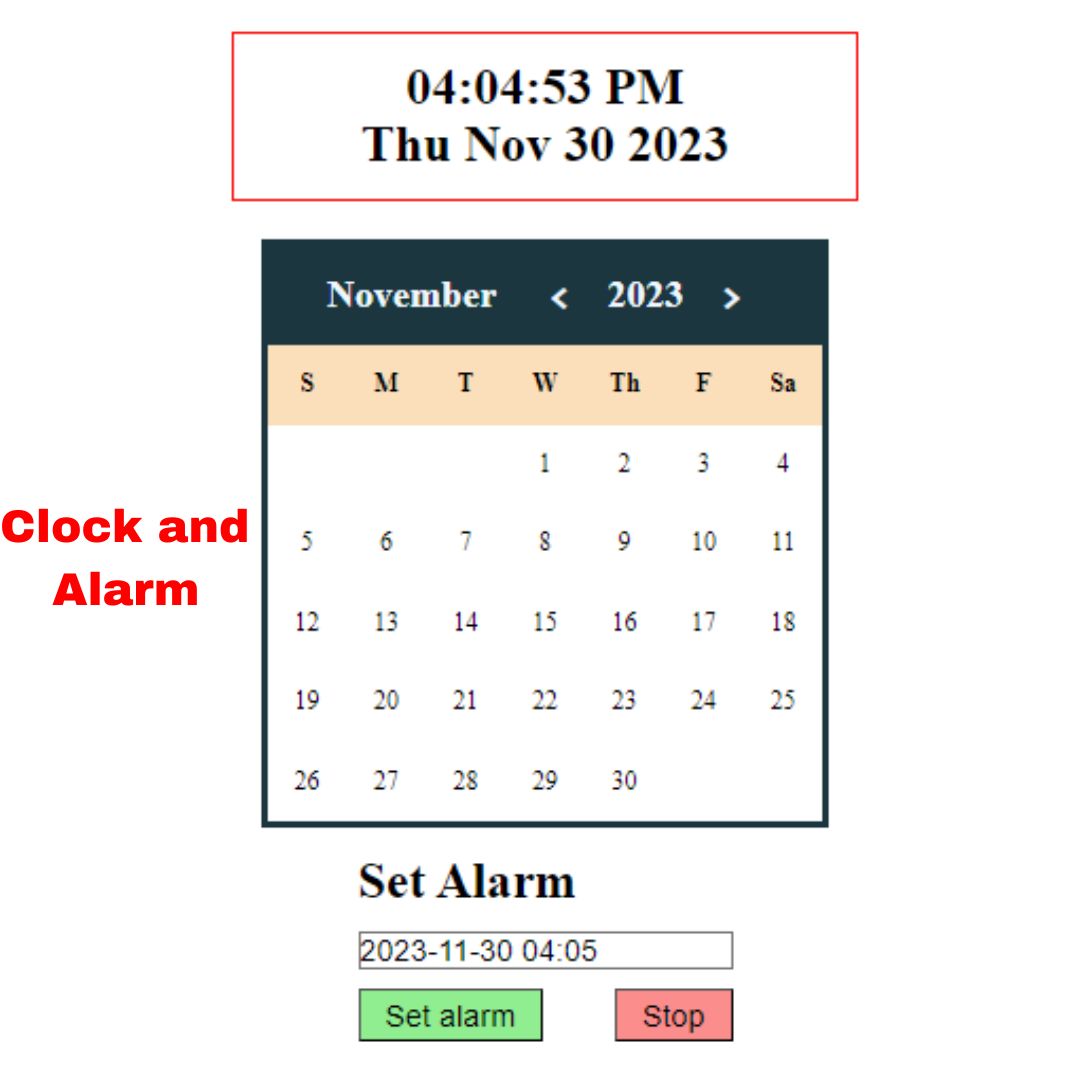
Crafting Custom Alarm and Clock Interfaces using HTML, CSS, and JavaScript
November 30, 2023
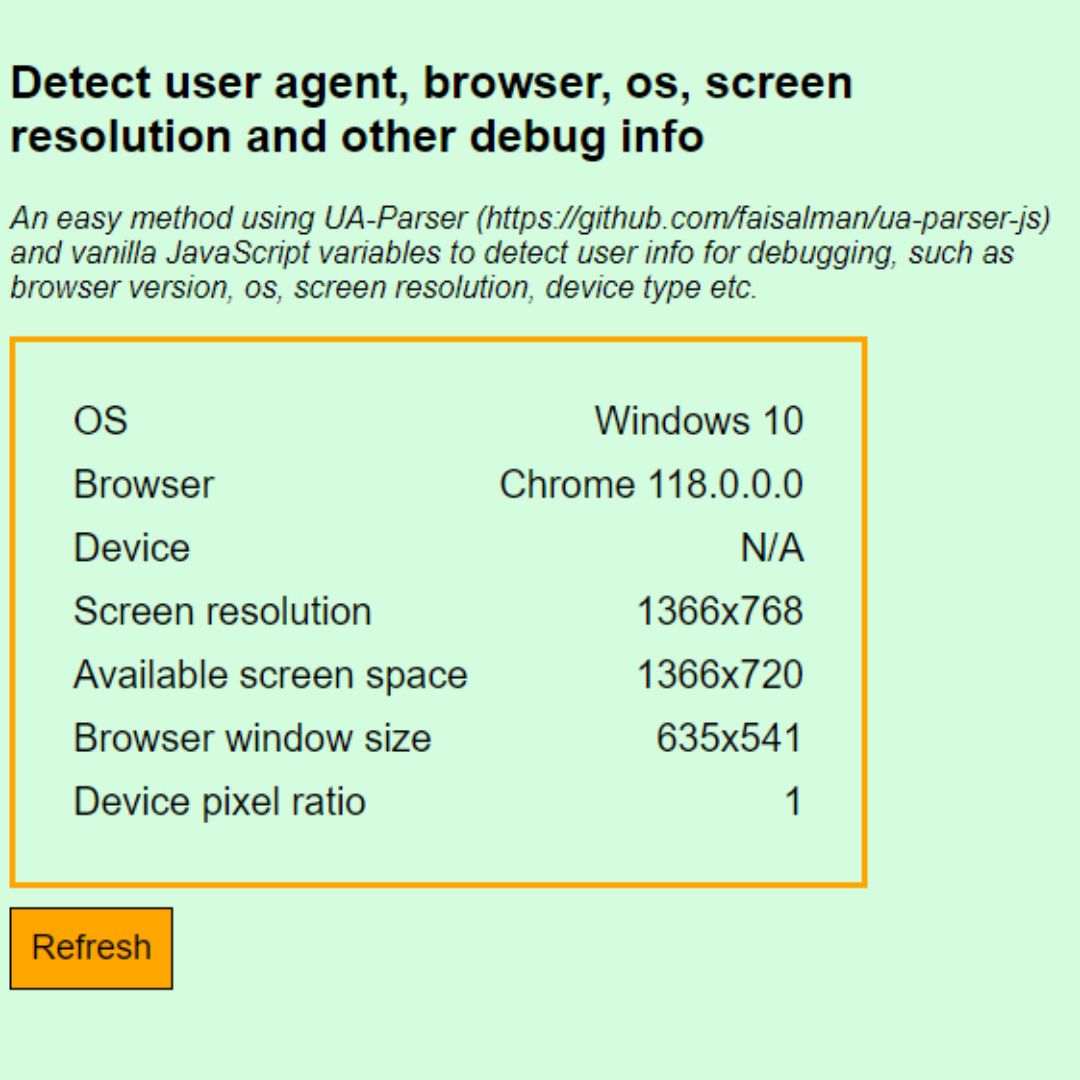
Detect User's Browser, Screen Resolution, OS, and More with JavaScript using UAParser.js Library
October 30, 2023
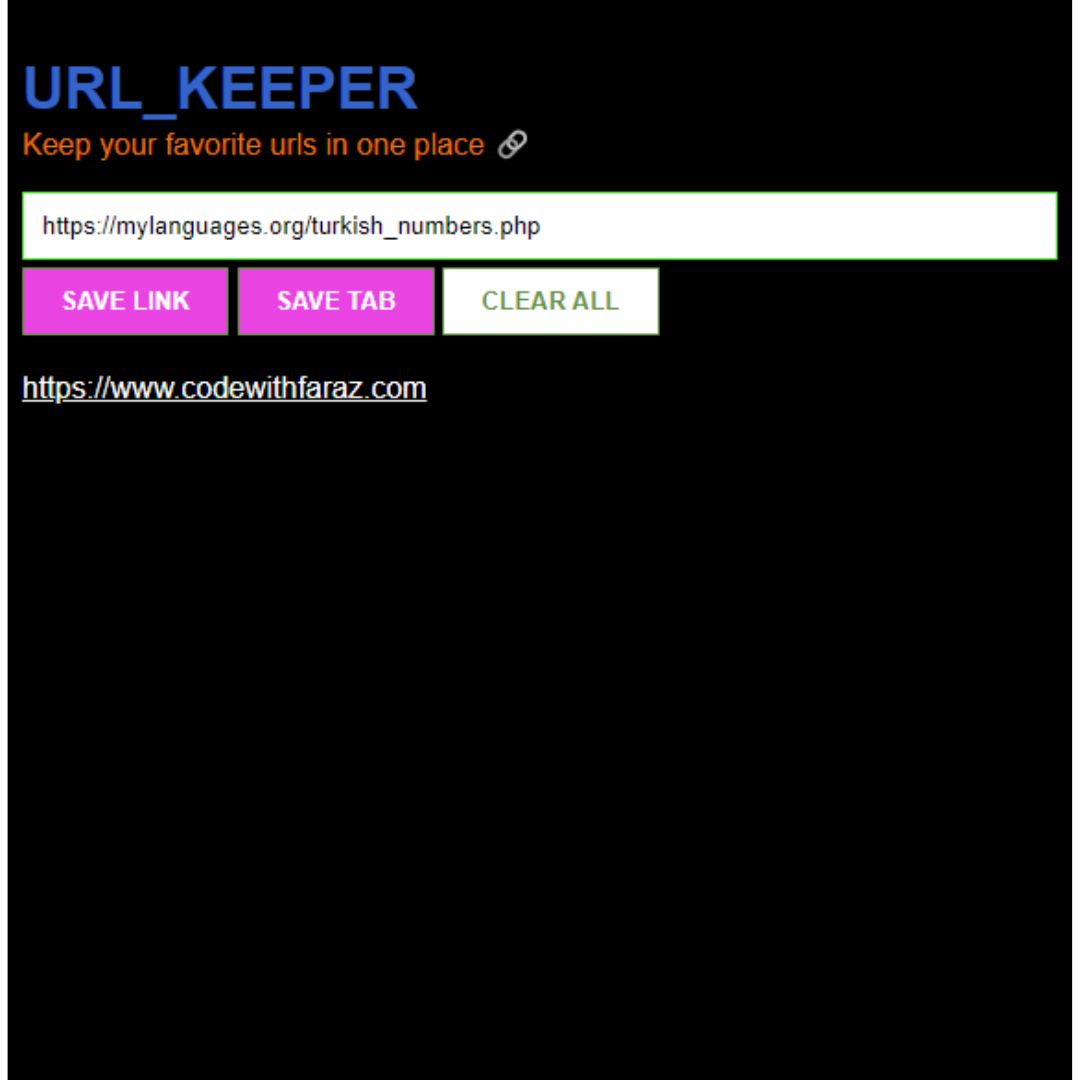
URL Keeper with HTML, CSS, and JavaScript (Source Code)
October 26, 2023
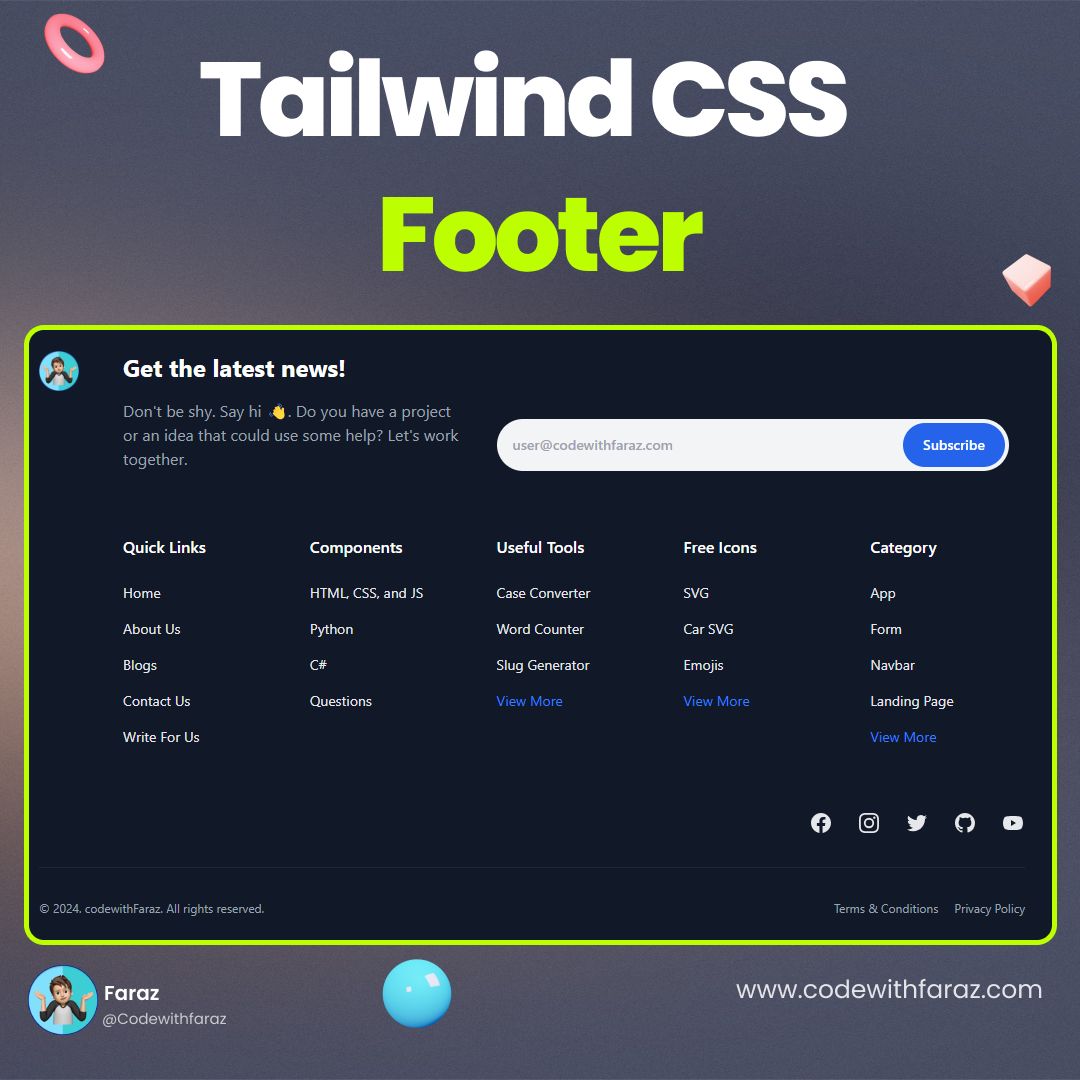
Creating a Responsive Footer with Tailwind CSS (Source Code)
Learn how to design a modern footer for your website using Tailwind CSS with our detailed tutorial. Perfect for beginners in web development.
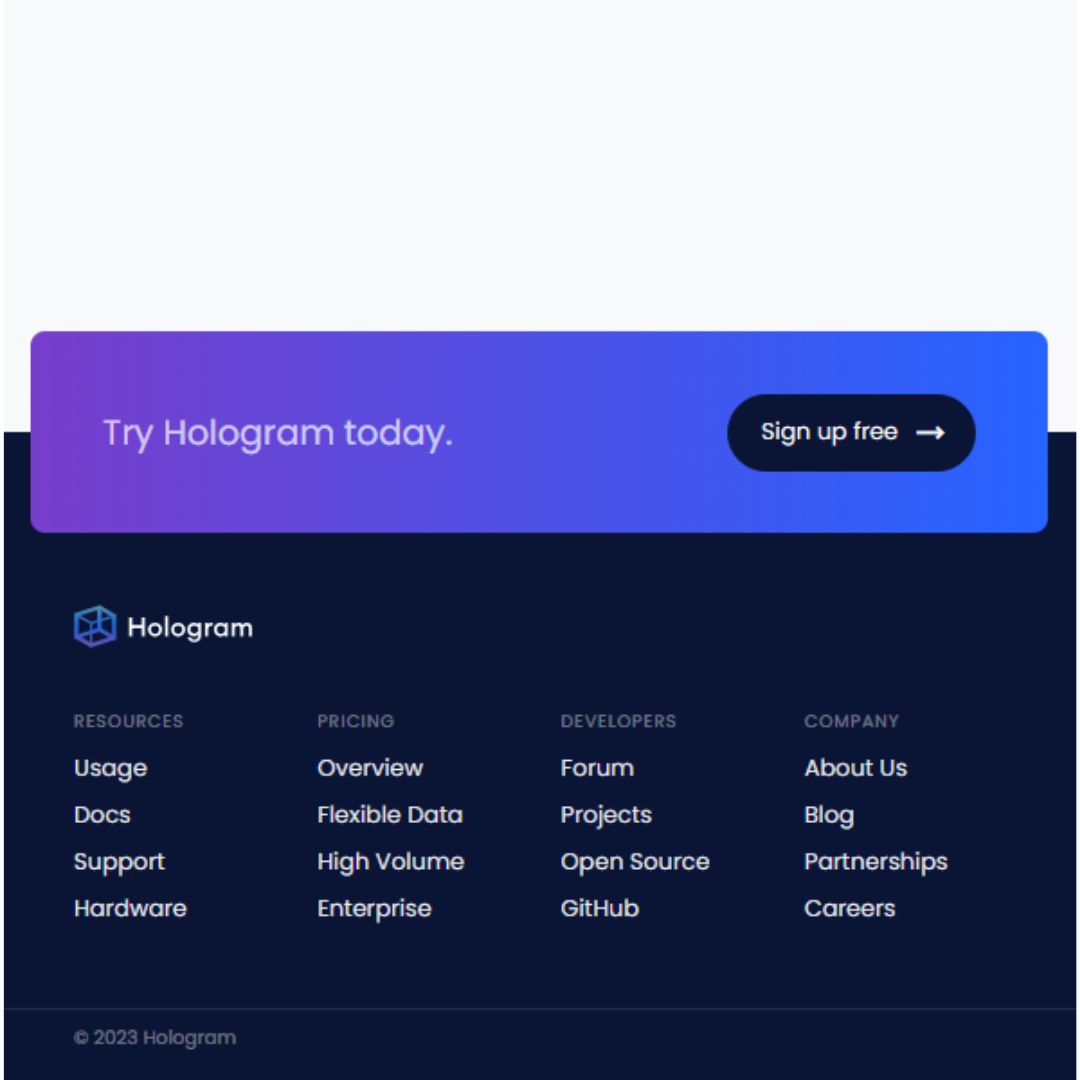
Crafting a Responsive HTML and CSS Footer (Source Code)
November 11, 2023
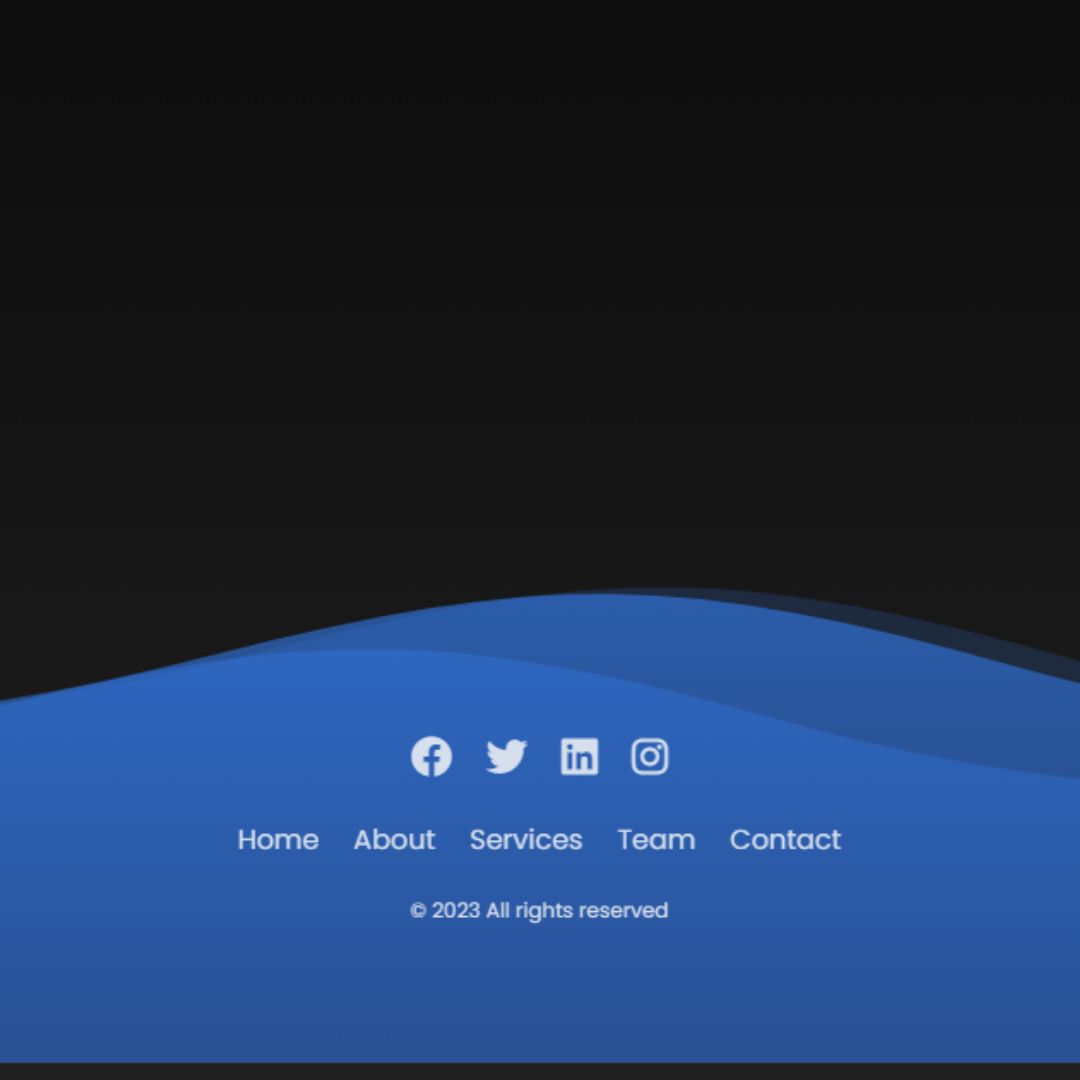
Create an Animated Footer with HTML and CSS (Source Code)
October 17, 2023
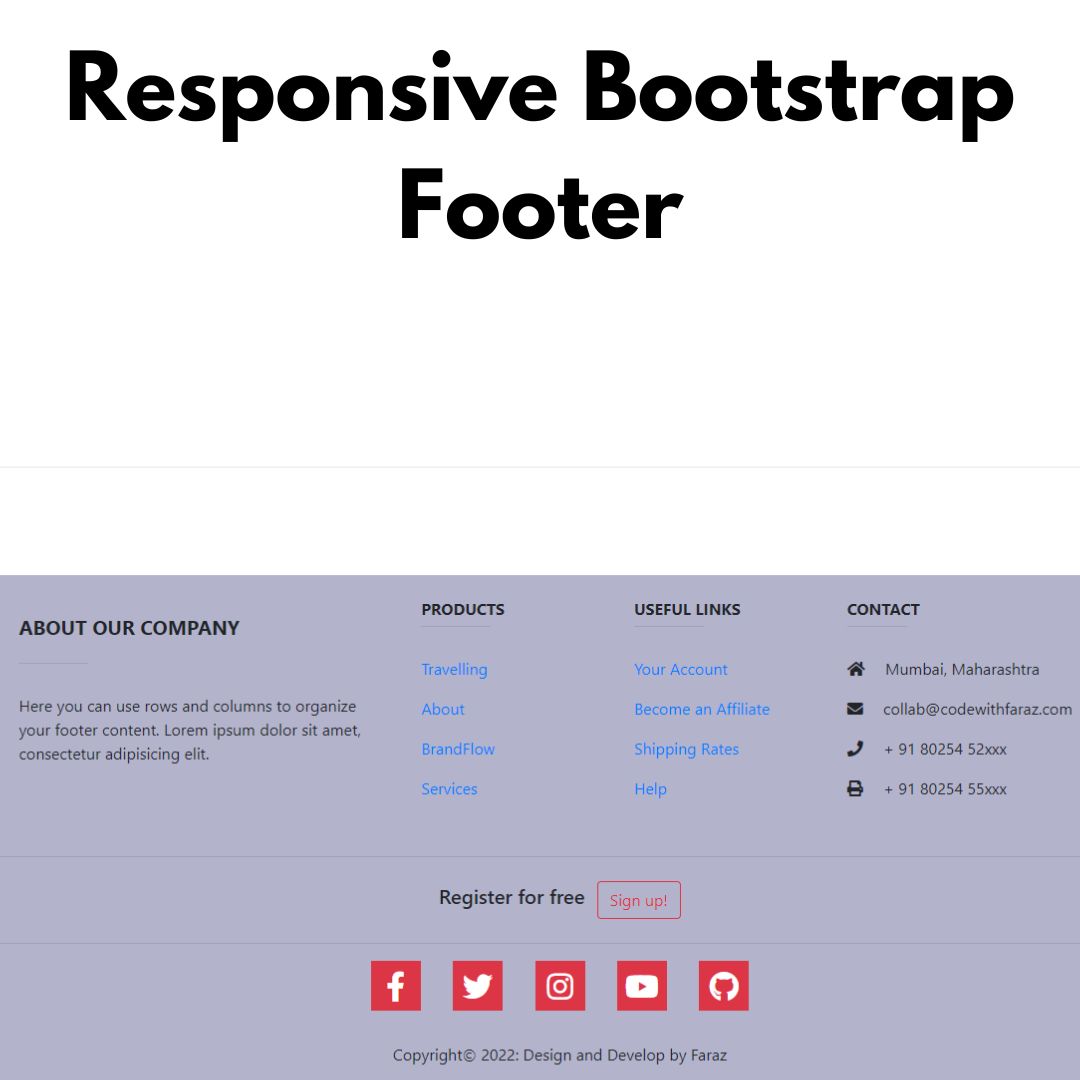
Bootstrap Footer Template for Every Website Style
March 08, 2023
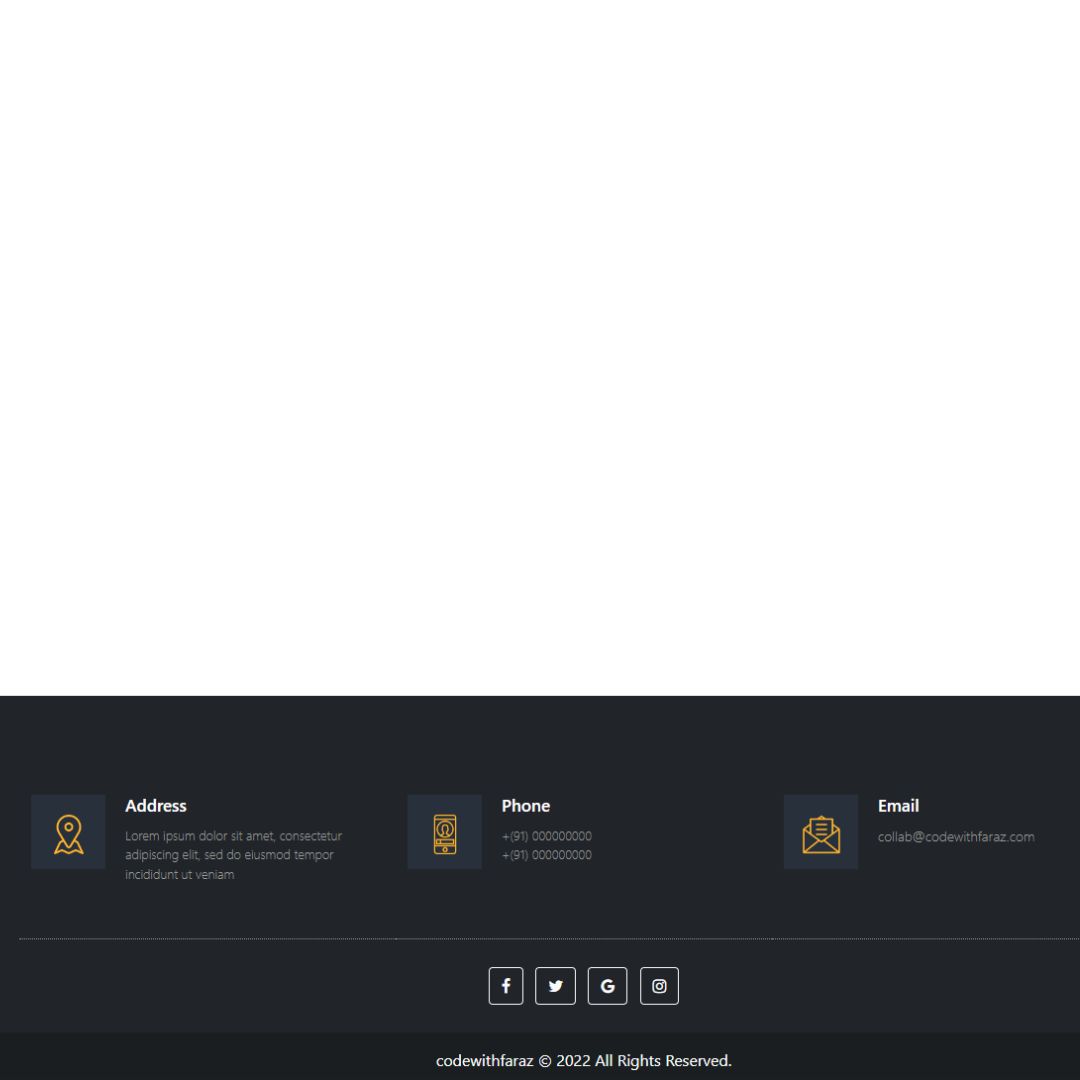
How to Create a Responsive Footer for Your Website with Bootstrap 5
August 19, 2022
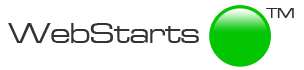
- 1-800-805-0920
Hit Counter
Simple Web Analytics
Pay when ready, open source, privacy-friendly, unique visitors.
Free Visitor Counter
Get your very own for your site. choose from six different styles in three easy steps., all counters 100% free, and without registration.
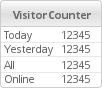
Select Color
Get your code.
Hit counter and visitor counter
The largest selection of free hit counter.
- Sign up free
- Changing web counter
- Chrome and Opera
A free web pages hit counters
No email needed for register your site and use the counter.
A counter customizable graphically. The biggest choice of counters. Create your web counter
All our counters are now in https (ssl) and asynchronous, you can use them in all https hosting.
If you wish, you can change your old codes, simply by adding the letter "S" at the end of http; Example: "http" > "https".
A wide range of styles
The most original web counters. Simple, fast and free of charge. Originals
Web applications
Custom creation.
You can not find your counter. Write us, and ask for custom counters Write Us
Latest graphic creations
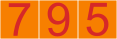
Hit 12 Counter web used
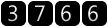
We are working to make it really useful tool, every day we add brand new counters for all tastes.
For users of the Chrome, Opera and Dragon Comodo browser.
The statistics in your browser bar. Free hit Counter applications for the Chrome and Opera browsers. In the Chrome Web Store & Store Opera is now offers applications for your web pages. See online of your web pages. Page extension : Google Chrome & Opera / Help chrome extension
The largest selection of hit counters
Our free hit counter and visitor counter is simple to install on your blog ( overblog , blogspot , blogger , tumblr ...) or website. Choose a visitor counter and install it in the html pages of your website or your blog. Click here to create the visit counter . The registration is free and No mail address needed. You can choose between a javascript counter or simple code html
Our statistics provide information about
- Monthly and daily changes in your traffic
- The number hit and number visitors of your site or blog
- Search engines and keywords
- See the backlink of your site
- Geolocation (country and city) of your visitors
- And many other data that you discover with free counter stat
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Design a simple counter using HTML CSS and JavaScript
- Design a Tip Calculator using HTML, CSS and JavaScript
- Age Calculator Design using HTML CSS and JavaScript
- Design a Nested Chat Comments using HTML CSS and JavaScript
- Design a Survey Form using HTML CSS and JavaScript
- How to Design a Simple Calendar using JavaScript ?
- Anagram Count using HTML CSS and JavaScript
- Create a Prime Number Finder using HTML CSS and JavaScript
- Create a Length Converter using HTML CSS and JavaScript
- Design Background color changer using HTML CSS and JavaScript
- Design Random Color Generator using HTML CSS and JavaScript
- Create a Pomodoro Timer using HTML CSS and JavaScript
- Design a Social Media Profile Card using HTML CSS & JavaScript
- Design a video slide animation effect using HTML CSS JavaScript
- Build a Memory Card Game Using HTML CSS and JavaScript
- Create a Pagination using HTML CSS and JavaScript
- Word and Character Counter using HTML CSS and JavaScript
- Create a Coin Flip using HTML, CSS & JavaScript
- Create an Online Art Auction using HTML, CSS and JavaScript
- How to make Incremental and Decremental counter using HTML, CSS and JavaScript ?
Counters are like those little number trackers you see on websites. You know, the ones that tell you how many likes or shares something has? They are pretty common on sites like online shops, social media, and games. This article is all about making one of those counters using JavaScript. We’ll go through how to do it step by step.
Here is the Sample Image of the counter that we are going to make:
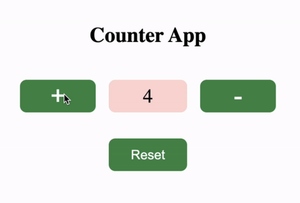
Preview Image
Before we start coding, let’s take a moment to understand the basic concepts behind a counter application. A counter is essentially a variable that keeps track of a numerical value. In JavaScript, we can create a variable using the ‘var’, ‘let’, or ‘const’ keywords . We will be using the ‘let’ keyword to create our counter variable, which can be incremented or decremented based on user actions. We will be also creating a button that will reset the counter.
Now, let’s get started with the coding part. We will be building a simple counter application that can be incremented, decremented, or can be reset using three different buttons. Here is the HTML code that we will be using:
HTML Code: File name – index.html
We have created a basic HTML structure with a heading and a div element containing two buttons and a div element that will display the counter value. We have also created a button for resetting the counter. We have also included a reference to our JavaScript file, ‘counter.js’ , using the ‘script’ tag.
Let’s style our counter app using CSS:
CSS Code: File name – style.css
Now, let’s move on to the JavaScript code. Here is the code that we will be using:
JavaScript Code: File name – counter.js
First, we have declared a ‘counter’ variable with an initial value of 0. We have also created four constants using the ‘const’ keyword to reference the HTML elements that we will be interacting with.
Next, we’ve added what are called event listeners to three buttons: ‘increment’, ‘decrement’, and ‘reset’.
When you click the ‘increment’ button, it adds 1 to the counter and shows the new number on the page.
When you click the ‘decrement’ button, it takes away 1 from the counter and shows the updated number.
And if you click the ‘reset’ button, it just sets the number back to zero.
So, to sum it up, counters are handy things on websites, and making one with JavaScript is pretty straightforward. With the code we’ve talked about in this article, you can get started on your own counter in no time. Just grasp the basics and use the code we’ve given you here.
Please Login to comment...
Similar reads.
- JavaScript-Projects
- Web Technologies
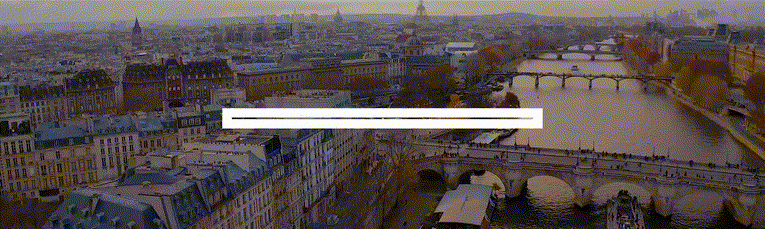
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Visitor Counter widget for website
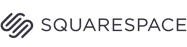
Why do I need Elfsight Visitor Counter?
Easy to analyze statistics, data in dynamics, increase trust level, join 2,078,753 customers already using elfsight apps, what makes elfsight visitor counter special.
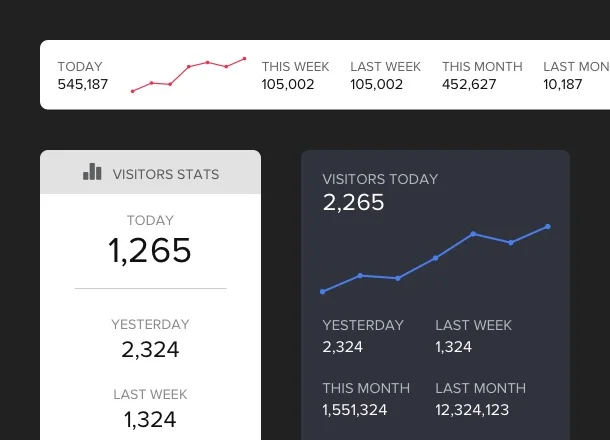
Why Elfsight widget of all others?
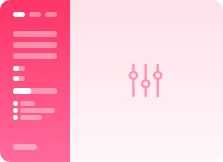
What our customers say ❤️
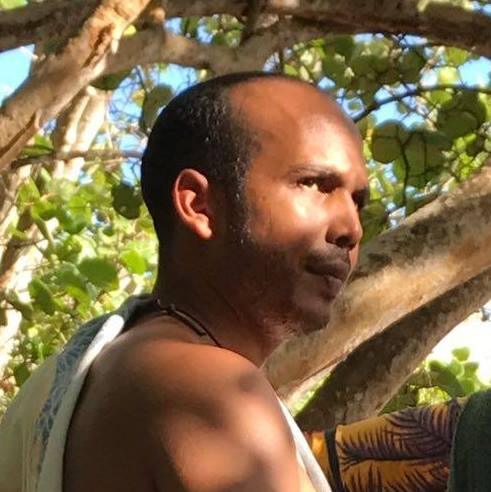
Frequently Asked Questions
What is a hit counter, which way do i get the widget’s code, how to add a visitor counter to a website.
To integrate our visitor widget, view the steps from the clear tutorial:
- Employ our demo and start shaping your custom plugin.
- Tailor the plugin aspects and apply the required edits.
- Receive the individual code which appears in the popup.
- Integrate the widget code into your website and save the page.
- You’ve properly integrated a new tool on your website.
Stumbled upon any problems during the installation? Address our support group and they will be glad to assist.
Does it go with my CMS or website builder?
Best way to use the visitor counter on your website, which way can i add a daily hit counter to my website, what info can i monitor with page view counter, how to build a website statistics graph by means of visitor counter widget, is there a way to transform the hit counter starting value.
Step into the Future: Say Goodbye to Outdated Analytics and Embrace Next-Gen Solutions!
Take Control of Your WordPress Analytics: Discover Advanced Page Visit Counter, the User-Friendly, High-Performance, doesn’t impact your site’s load time, and GDPR-Compliant Plugin!
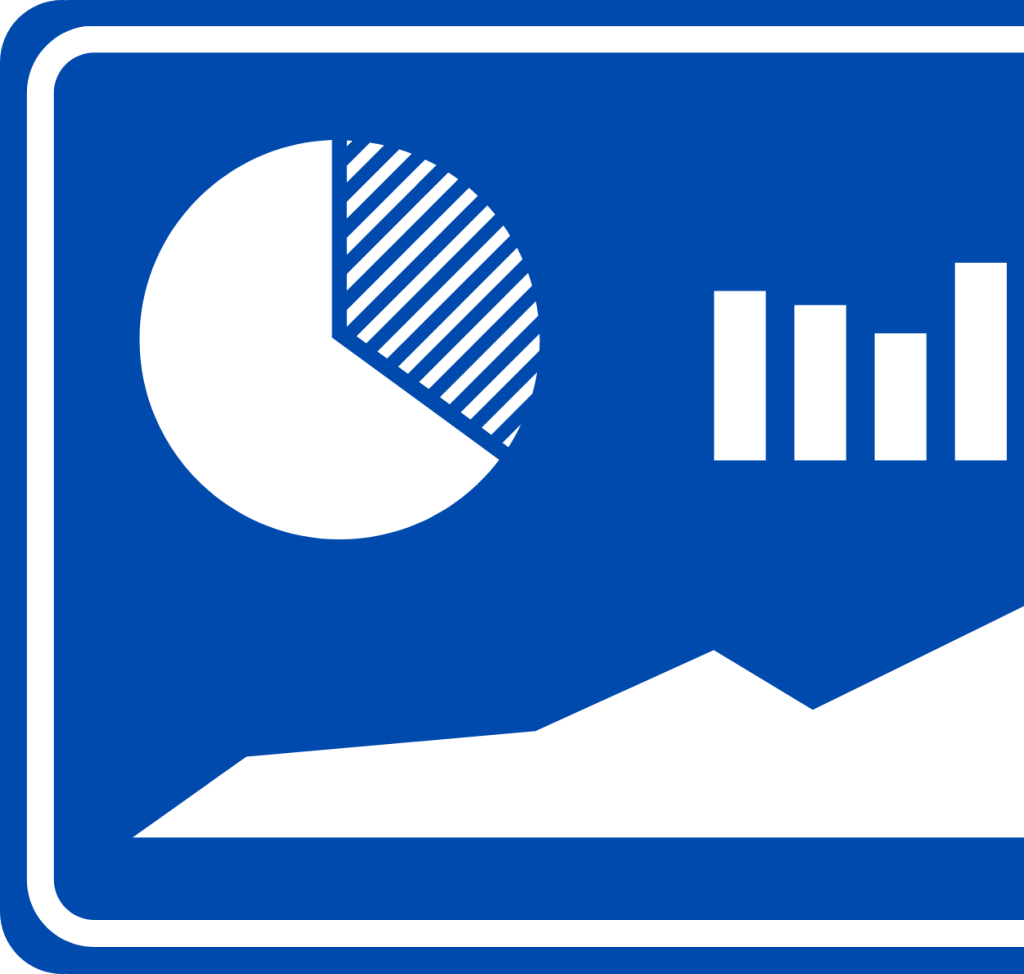
Discover All the Available Features
Made for wordpress.
Seamless integration with WordPress, eliminating the need for external accounts.
Insightful analytics dashboard
Enhance your WordPress site with a fully informative analytics dashboard.
100% GDPR compliant
With our solution, you can rest assured that your analytics activities are fully aligned with GDPR regulations.
Sources of website traffic
Discover the websites that are driving the highest number of monthly visitors to your site.
Simple and effortless setup
Begins tracking instantly upon installation, requiring no additional configuration.
Campaign Builder
Generate and monitor personalized campaign URLs effortlessly. With premium version only.
Real-time Analytics
Observe the current number of visitors on your website in real-time and identify their geographical locations.
WooCommerce Analytics
Get valuable insights into the performance of your WooCommerce store through robust analytics.
Data Export to CSV File
Effortlessly export your data to a CSV file for seamless analysis and reporting.
Shortcode Generator
This plugin is equipped with a powerful and extensive shortcode generator tool.
Exciting new features will be released soon, offering even more functionality.
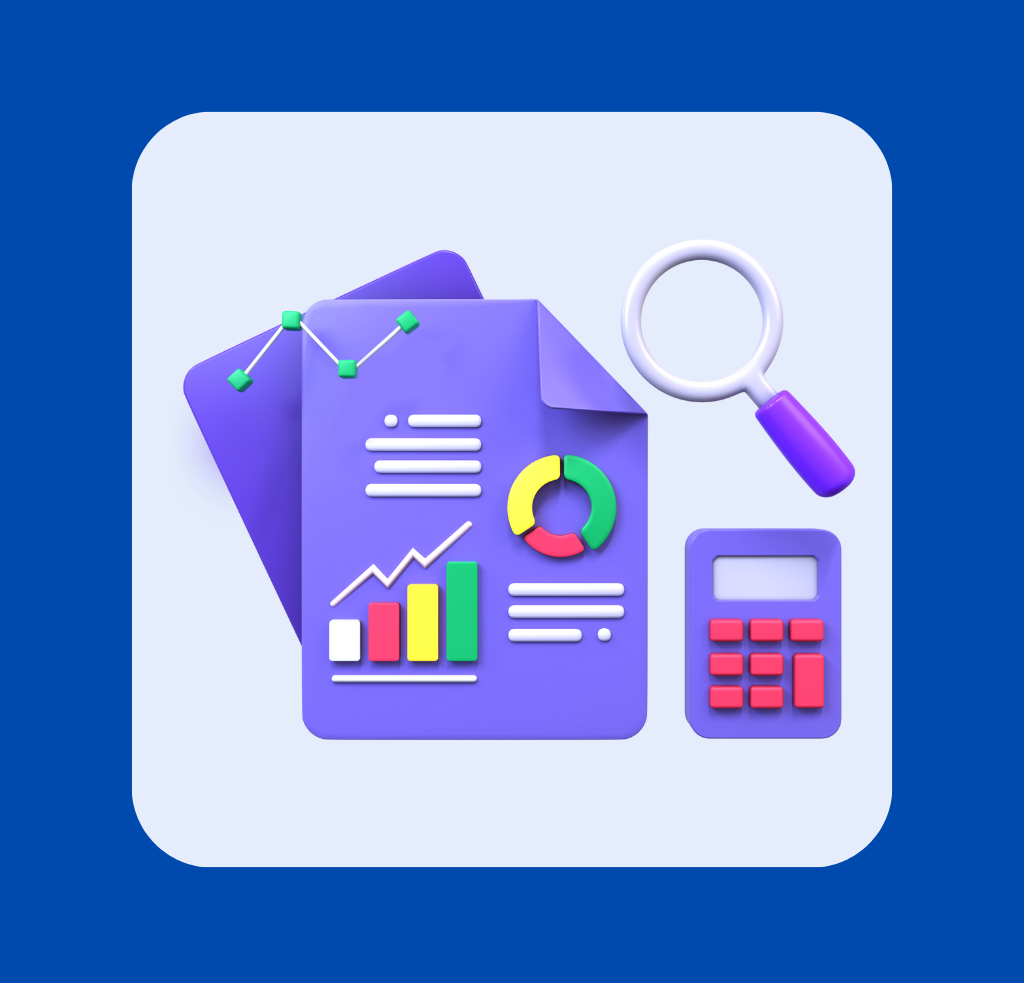
Amazing Dashboard
Take your WordPress site to the next level with a comprehensive and informative analytics dashboard that provides valuable insights and data-driven metrics.
Gain in-depth analytics for your pages, posts, users, user sessions, and page views with an interactive chart that includes a date range filter for precise data analysis.
Trending Data
Trending analytics is a powerful tool that allows organizations to stay ahead of the curve by identifying and leveraging emerging trends in their data. By analyzing patterns, correlations, and shifts in various metrics, trending analytics helps uncover valuable insights that can drive strategic decision-making and business growth.
This module provides comprehensive analytics on various aspects including the top 10 pages, top 10 posts, top 10 countries, top 10 states, top 10 cities, top 10 devices, top 10 operating systems, and top 10 browsers, offering detailed insights into user behavior and demographics.
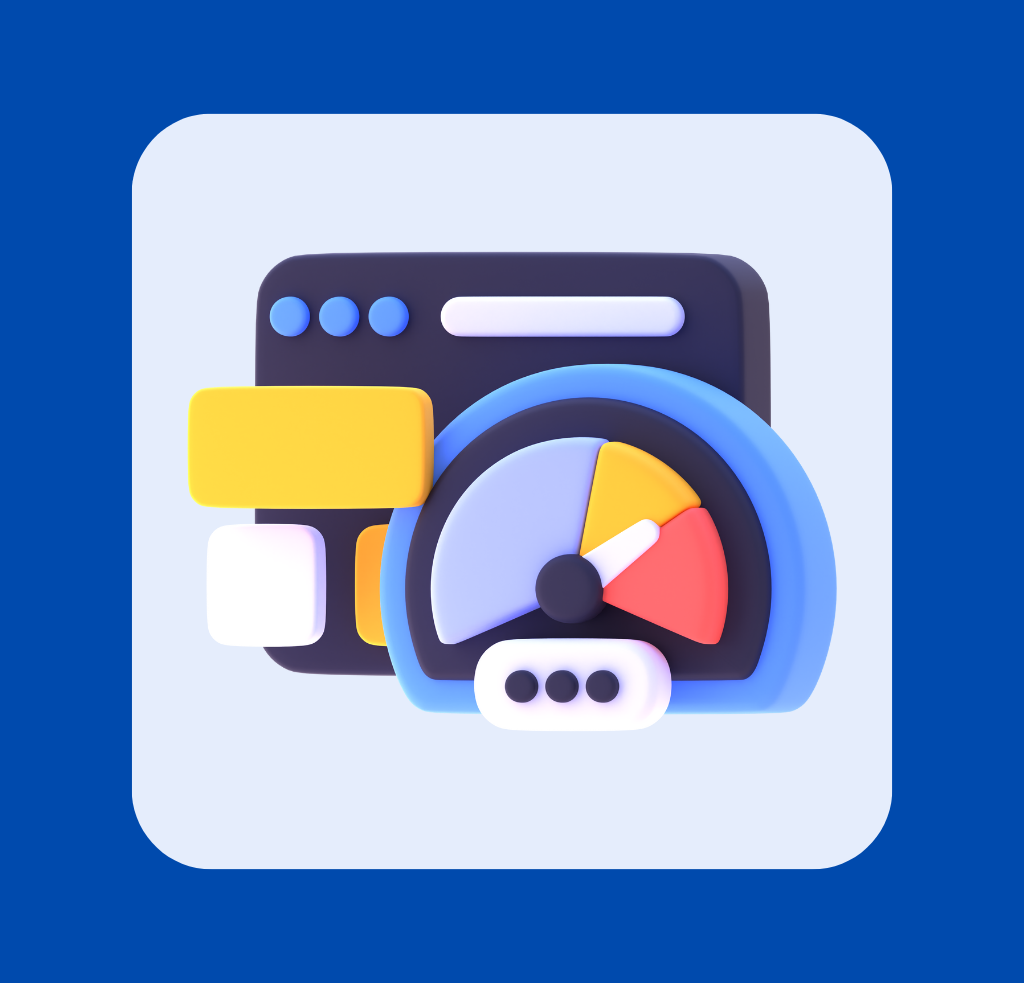
Real-time stats
Real-time visitors refer to the individuals who are actively accessing and engaging with a website or online platform at any given moment. Real-time visitor tracking provides valuable insights into the current activity and behavior of users on a website, offering businesses a glimpse into the immediate impact of their online presence.
- Real-time User's location
- Real-time updates about pages
- Real-time campaign and referrers data
Campaigns play a pivotal role in marketing strategies, serving as targeted and focused initiatives designed to achieve specific goals. Whether it’s promoting a new product, increasing brand awareness, driving website traffic, or generating leads, campaigns provide a structured approach to reaching your desired outcomes.
This module offers a range of options to create campaign URLs and conveniently track them at a later stage.
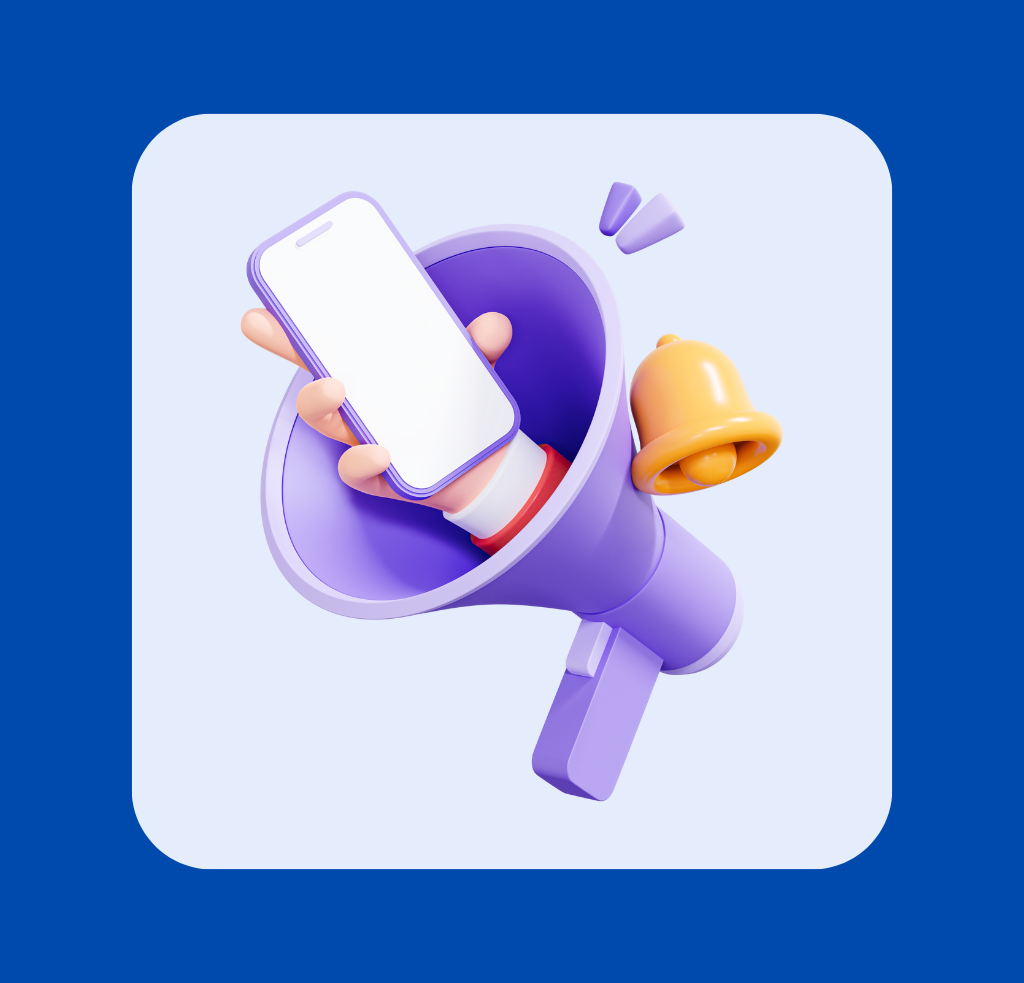
WooCommerce Stats
WooCommerce Statistics provide valuable insights into the health and success of an online store, allowing businesses to make data-driven decisions and optimize their operations. Some key areas of analysis include:
- Sales Performance
- Product Performance
- Customer Behavior
- Traffic Sources
Export Data
With the export feature, you can easily generate a CSV file containing comprehensive data, including user details, such as location, number of pages visited, and various other details. This export functionality allows for convenient analysis and further processing of the data outside of the WooCommerce platform.
- Include selected post types
- Geo-graphic data
- Device data
- Campaign data
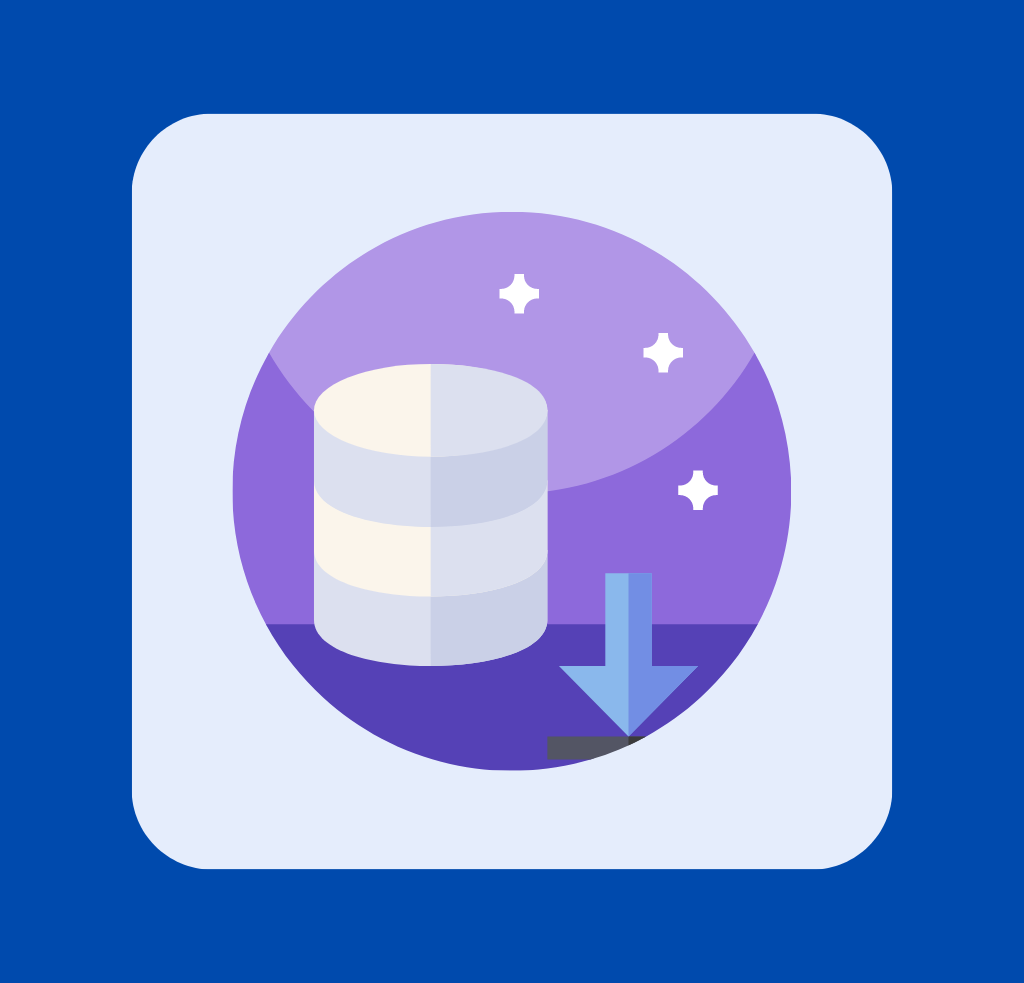
Active sites
Premium Users
Choose the right pricing plan for you
$ 129.9 / Yearly
- Everything from essential
- 5 sites licenses
$ 49.9 / Yearly
- Single Site License
- Informative dashboard analytics
- Simple WordPress integration
- Ensures optimal site performance
- Real-time statistics
- Trending data
- Referrers data
- Shortcode generator tool
- Smart Campaigns tool
- Export data tool
- 1 year priority support
- Weekly and Bi-weekly updates
$ 329.9 / Yearly
- Unlimited sites licenses
- 24x7 Priority support
$ 389.9 / Lifetime
$ 149.9 / Lifetime
- Lifetime 24x7 Priority support
$ 989.9 / Lifetime
We have the trust of thousands of users.
Very informative and i review every day useful item use it daily to see the activity of my site.
Vary Useful tool use it daily. Keeps track of the people to visit my site that I can see what works and what doesn’t
Reginald Gossert
Extremely easy and neat
Renato Deformes
Get the latest information from us.
CSS Tutorial
Css advanced, css responsive, css examples, css references, css counters.
CSS counters are "variables" maintained by CSS whose values can be incremented by CSS rules (to track how many times they are used). Counters let you adjust the appearance of content based on its placement in the document.
Automatic Numbering With Counters
CSS counters are like "variables". The variable values can be incremented by CSS rules (which will track how many times they are used).
To work with CSS counters we will use the following properties:
- counter-reset - Creates or resets a counter
- counter-increment - Increments a counter value
- content - Inserts generated content
- counter() or counters() function - Adds the value of a counter to an element
To use a CSS counter, it must first be created with counter-reset .
The following example creates a counter for the page (in the body selector), then increments the counter value for each <h2> element and adds "Section < value of the counter >:" to the beginning of each <h2> element:
Advertisement
Nesting Counters
The following example creates one counter for the page (section) and one counter for each <h1> element (subsection). The "section" counter will be counted for each <h1> element with "Section < value of the section counter >.", and the "subsection" counter will be counted for each <h2> element with "< value of the section counter >.< value of the subsection counter >":
A counter can also be useful to make outlined lists because a new instance of a counter is automatically created in child elements. Here we use the counters() function to insert a string between different levels of nested counters:
CSS Counter Properties

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Purdue Online Writing Lab Purdue OWL® College of Liberal Arts
Welcome to the Purdue Online Writing Lab
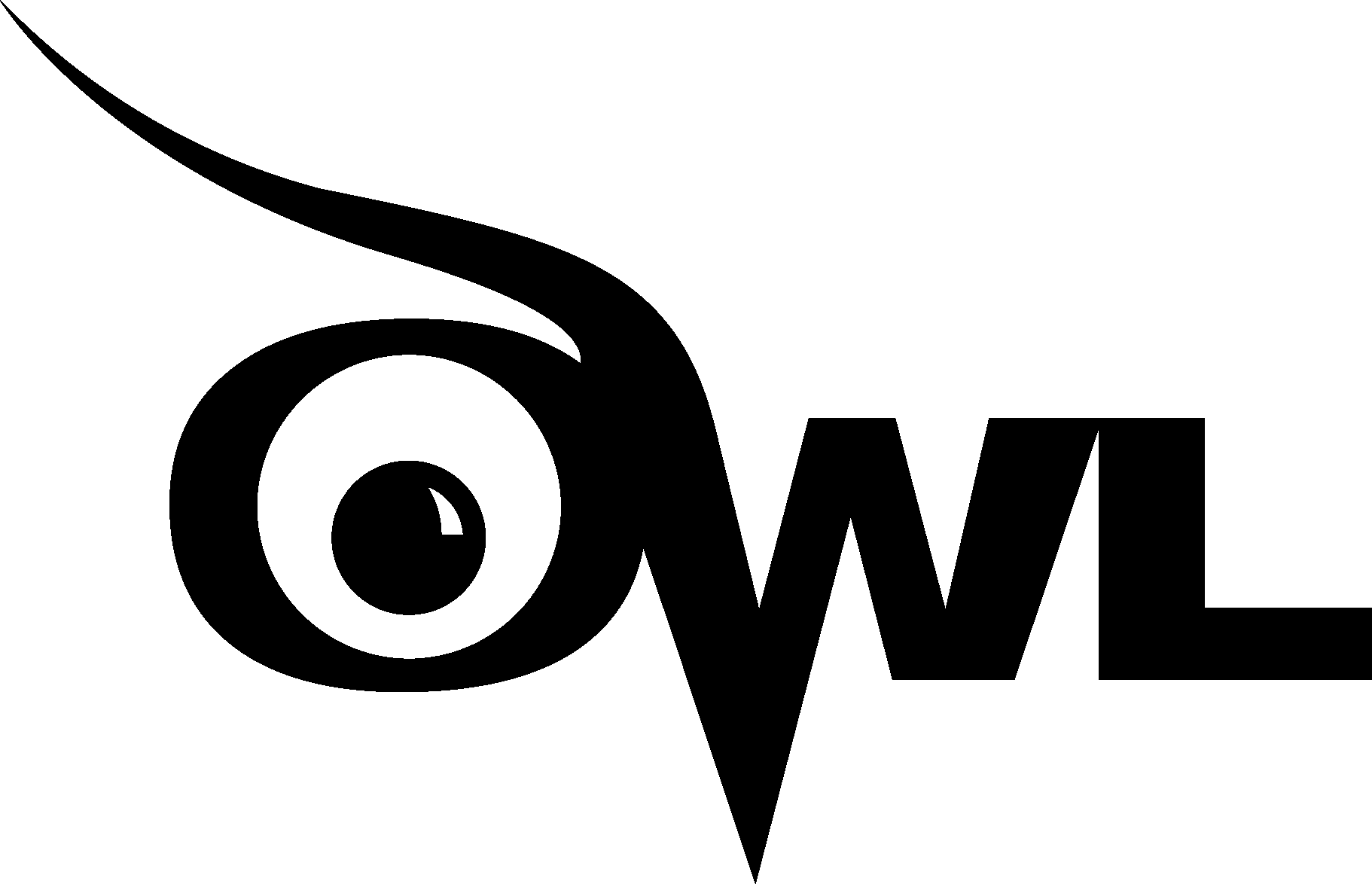
Welcome to the Purdue OWL
This page is brought to you by the OWL at Purdue University. When printing this page, you must include the entire legal notice.
Copyright ©1995-2018 by The Writing Lab & The OWL at Purdue and Purdue University. All rights reserved. This material may not be published, reproduced, broadcast, rewritten, or redistributed without permission. Use of this site constitutes acceptance of our terms and conditions of fair use.
The Online Writing Lab at Purdue University houses writing resources and instructional material, and we provide these as a free service of the Writing Lab at Purdue. Students, members of the community, and users worldwide will find information to assist with many writing projects. Teachers and trainers may use this material for in-class and out-of-class instruction.
The Purdue On-Campus Writing Lab and Purdue Online Writing Lab assist clients in their development as writers—no matter what their skill level—with on-campus consultations, online participation, and community engagement. The Purdue Writing Lab serves the Purdue, West Lafayette, campus and coordinates with local literacy initiatives. The Purdue OWL offers global support through online reference materials and services.
A Message From the Assistant Director of Content Development
The Purdue OWL® is committed to supporting students, instructors, and writers by offering a wide range of resources that are developed and revised with them in mind. To do this, the OWL team is always exploring possibilties for a better design, allowing accessibility and user experience to guide our process. As the OWL undergoes some changes, we welcome your feedback and suggestions by email at any time.
Please don't hesitate to contact us via our contact page if you have any questions or comments.
All the best,
Social Media
Facebook twitter.
- Cast & crew
Alien: Romulus

While scavenging the deep ends of a derelict space station, a group of young space colonizers come face to face with the most terrifying life form in the universe. While scavenging the deep ends of a derelict space station, a group of young space colonizers come face to face with the most terrifying life form in the universe. While scavenging the deep ends of a derelict space station, a group of young space colonizers come face to face with the most terrifying life form in the universe.
- Fede Alvarez
- Rodo Sayagues
- Dan O'Bannon
- Cailee Spaeny
- Isabela Merced
- Archie Renaux

- Rain Carradine

- All cast & crew
- Production, box office & more at IMDbPro
The Big List of Summer Movies

More like this

Did you know
- Trivia Director Fede Alvarez sought out special effects crew from Aliens (1986) to work on the creatures. Physical sets, practical creatures, and miniatures were used wherever possible to help ground later VFX work.
- Connections Referenced in That Star Wars Girl: New Alien Series Won't Have Ellen Ripley Will Instead Be About Human Class Inequality (2021)
- When will Alien: Romulus be released? Powered by Alexa
- August 16, 2024 (United States)
- United Kingdom
- United States
- Quái Vật Không Gian: Romulus
- Origo Studios, Budapest, Hungary
- 20th Century Studios
- Scott Free Productions
- Brandywine Productions
- See more company credits at IMDbPro
Technical specs
- Dolby Atmos
Related news
Contribute to this page.

- See more gaps
- Learn more about contributing
More to explore

Recently viewed
Language selection
- Français fr
CSIS Act Amendments: Bolstering Canada’s counter-foreign interference legislation
From: Public Safety Canada
Backgrounder
The Government of Canada introduces legislation to bolster Canada’s response to foreign interference.
Foreign interference is one of the greatest strategic threats facing Canada’s sovereignty and democracy. It threatens all orders of government, the private sector, media, academia, communities and individuals in Canada. Foreign interference attempts to erode our democratic processes and institutions, interferes in our economy and critical sectors and threatens Canadian communities.
The Canadian Security Intelligence Service (CSIS) is mandated to detect, and when appropriate, take action to disrupt threats, including those posed by foreign interference. The nature of these threats continues to evolve over time, so updating the framework to address modern challenges is crucial and will bring benefits.
The CSIS Act was enacted in 1984 at a time when the prolific use and expansion of digital technology could not have been foreseen. Today, digital technologies permeate every aspect of life in Canada. Electronic information and data have become a large and important part of national security investigations but key, and often basic, pieces of information are no longer accessible through conventional investigative techniques. Countering the foreign interference threat that Canada faces today requires urgent updates to the CSIS Act to better equip CSIS to carry out its mandate to investigate, advise the Government of Canada, and take measures to reduce this strategic threat to the security of Canada.
Moreover, the targets of hostile states and threat actors have shifted beyond the Government of Canada, to partners in academia, private industry, provincial, territorial, and Indigenous governments, and community groups. These entities often lack access to critical government information that would help them build resiliency against such threats. Investigating the foreign interference and other threats that Canada faces today requires that CSIS be able to equip national security partners, operate in a digital world, and respond to evolving threats.
Consultations with the Canadian public
CSIS and Public Safety held extensive online, virtual and in-person consultations on amending the CSIS Act from November 24, 2023, to February 2, 2024. A diverse range of stakeholders, including representatives from provincial, territorial and Indigenous governments and partners, academia, the business community, as well as community representatives were engaged in this context. These consultations provided people from across the country with an opportunity to share their views on potential amendments to the CSIS Act . The valuable input and advice gathered were instrumental in shaping the drafting of this legislation.
During consultations, participants noted that advancements in technology and evolving threats have created a need for updates to the CSIS Act, and looked favourably at changes that would better equip CSIS and the Government of Canada to respond to national security threats such as foreign interference. Respondents held a generally positive view toward the need for amendments across each of the categories under consideration. The desire for CSIS to share more information emerged as a prominent theme. Stakeholders, including representatives from provincial, territorial, municipal and Indigenous governments and partners, often expressed that receiving information from CSIS would help them better understand, recognize and build resilience against foreign interference.
While overall support was strong, not all participants favoured all the proposals. A minority of respondents expressed concerns with respect to privacy, and the need for strong oversight and accountability. They also noted the importance of continuing to strengthen trust in CSIS as an institution and ensuring continued efforts by CSIS to increase transparency. Finally, respondents frequently noted the need for Canada to be stronger in the face of national security threats like foreign interference.
The What We Heard and Learned Report has been posted on the Consultation webpage to provide greater transparency to the Canadian public on the extensive consultations that were undertaken to inform the policy development and drafting of legislative amendments.
Proposed legislative amendments
Targeted amendments to modernize the CSIS Act would better equip the Government of Canada to build resilience and to counter the modern threats Canada faces today, including foreign interference.
To ensure the safety, security, and prosperity of Canada, CSIS must be able to:
Equip national security partners
- This legislation would enable broader disclosure of CSIS information to key partners beyond the Government of Canada, with appropriate safeguards, to help partners build resiliency to threats.
- Amendments would help partners build resiliency to threats by helping them to better identify and integrate proactive measures.
Operate in a digital world
- The absence of tailored judicial warrants, impedes, delays, and at times altogether halts national security investigations, diminishing CSIS’ ability to protect all Canadians.
- This legislation would increase CSIS’ ability to be more agile and effective in its investigations, by introducing new warrants for specific investigative techniques.
- It would also enhance CSIS’ capacity to collect and use datasets.
- Amendments would close the gap created by technology as well as regain the ability for CSIS to collect, from within Canada, foreign intelligence about the intentions and capabilities of foreign states and foreign individuals in Canada.
Respond to evolving threats
- This legislation would implement a statutory requirement for Parliament to periodically review the CSIS Act to ensure that it keeps pace with advances in technology and data, as well as other evolutions in the national security space.
Accountability and Transparency
The authorities in the CSIS Act must reflect the values and ideals of the Canadian public, whom CSIS seeks to protect. Informed by the consultations, these amendments have been developed considering the high expectation of privacy that people in Canada have, including the protection provided by the Canadian Charter of Rights and Freedoms implications as core considerations.
The amendments maintain existing systems of review, oversight, and transparency with appropriate safeguards, including for all new powers. CSIS has multiple layers of protections to ensure that CSIS is accountable and that the rights of people in Canada are respected. For instance, CSIS is required to seek a Federal Court warrant before undertaking activities that are more than minimally intrusive. CSIS would continue to require the approval of the Federal Court for all warrants.
The National Security and Intelligence Review Agency and the National Security and Intelligence Committee of Parliamentarians also provide an important review function for CSIS’ activities. Certain activities, such as collection and retention of datasets, are also subject to the review and approval of the Intelligence Commissioner.
Finally, CSIS is accountable to the Canadian public and is committed to increasing transparency to ensure that they have trust in their security intelligence service. CSIS continues to listen to the needs and concerns of the communities it seeks to protect, and works to maintain this trust to effectively meet its mandate. With these amendments, the Government of Canada will be better equipped to protect Canada and the Canadian public from foreign interference and other threats.
Page details
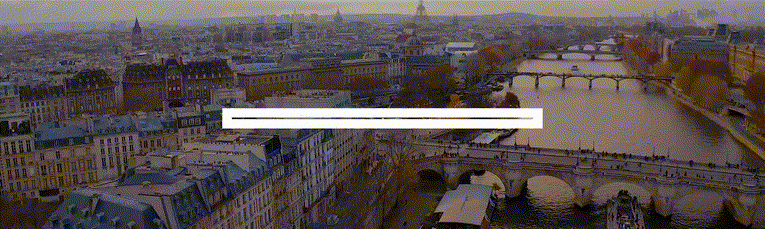
IMAGES
VIDEO
COMMENTS
I need a counter to integrate inside my HTML code that counts from one to three when the visitors visited my webpage. For example if the first visitor visited my page it count to 1, then the next visitor visited the page it count to 2, and for the 3rd visitor count to 3, then for the 4th visitor again starts from 1 and so on.
visitCount = 1; //Add entry for key="page_view" localStorage.setItem("page_view", 1); 5. Increment visitor count and update the localStorage. On the contrary previous scenario, if an entry for the "page_view" key is already present, the retrieved String value is converted to a number datatype using Number(). The previous session visitCount is then incremented and the value is updated in ...
To create a counter, you must create a Perl, PHP (PHP: Hypertext Preprocessor), or another script. Then, either refer to that script through a server-side include or another method. We recommend you already know or learn Perl or PHP programming to create the counter script. The code for creating a counter can vary, depending on the programming ...
var counter = 001184; var newnum = 001184 + 1; var el = document.getElementsByClassName("counter-item"); el.innerHTML = newnum; This is how the result will look like when i refresh the browser, but it is static for now.
Step 6: Manually check the counter Now I'm goin to teach you how to check the number of visits on your website without having to actually visit your website. This can be useful for three reasons.
Step 2 (CSS Code): Once the basic HTML structure of the counter is in place, the next step is to add styling to the counter using CSS. Next, we will create our CSS file. In this file, we will use some basic CSS rules to style our counter.
3.8m Websites Built & Hosted. Create a free, custom hit counter for your website. Choose from multiple styles. Generate HTML code and embed it on any website. See how many people have visited your website or web page.
Multiple people can visit your site from the same browser (makes cookies useless) The closest you can get is to store the visitors IP in combination with a cookie to not count those in the future. Here is a tradeoff, if they clear the cookie, they are a new visitor. If you only store the IP, you count whole proxies as one visitor.
Take note of the singular button element that is within the body of the document. As it stands, this will create an HTML page with just a single small button on it. On the sample CSS code below the "counter-reset" is added within the body of the HTML document. body { counter-reset: " Name of the HTML counter ";}
Login to your blogger account (opens in new window). Click Layout in left menu. Click the "Add a Gadget" link where you want to add the code (at the bottom is best) Scroll down and click the plus next to "HTML/JavaScript" in the popup window. Enter 'StatCounter' in the title box. Paste the code in the content box. Click 'save'.
Pay when ready. Track website visitors with Counter, a privacy-friendly analytics tool. Get daily unique visitor counts, referrer information, and geographical locations. Our open source web analytics tool makes it easy to understand your web traffic. Get started today and pay what and when you want!
Your Free Hit Counter. - free of charge, cost-free, 100% free! - use without registration. - easy to embed on every homepage or website. - counter is usable with Wordpress and easily embeddable. - simply change the counter style by changing the style number in the code (warning: do not change anything else!)
The largest selection of hit counters. Our free hit counter and visitor counter is simple to install on your blog ( overblog , blogspot , blogger , tumblr ...) or website. Choose a visitor counter and install it in the html pages of your website or your blog. Click here to create the visit counter . The registration is free and No mail address ...
Hit counter | Get Free visitor Counter for your website. HIT COUNTER. No sign-up or registration! Enter the domain or URL, select the number you want to begin from, the number of digits, counter type and style and click the big blue button. The HTML code for your counter will be ready in no time.
We have also included a reference to our JavaScript file, 'counter.js', using the 'script' tag. Let's style our counter app using CSS: CSS Code: File name - style.css. Now, let's move on to the JavaScript code. Here is the code that we will be using: JavaScript Code: File name - counter.js.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... Section Counter. A section counter is used to tell people how well their business is going, by displaying interesting numbers: 11+ Partners. 55+ Projects. 100+
Free Hit Counter for WebSites and Blogs. Just place the HTML code on your website. The number is displayed with image. There are 152 styles to choose from. A web counter or hit counter or visitor counter is a computer software program that indicates the number of visitors, or hits, a particular webpage has received.
VISITOR HIT COUNTER. Adding a hit counter will enable you and users to keep an eye on visits and page views of the site. You can create one in the tool by choosing the number of digits and where you desire the counter should start counting. Click on "Generate Counter HTML code" and embed at a suitable location on your website.
Create all types of popups: banners, bars, notifications and more for any purpose. Best seller. Create your custom Visitor Counter widget for the website in a few clicks. Try a free demo for shaping Visitor Counter by Elfsight.
Single Site Licenses. $49.9/Yearly. Below are some incredible features available with the premium subscription: Single Site License. Zero setup. Informative dashboard analytics. Simple WordPress integration. Ensures optimal site performance. Real-time statistics.
You can apply CSS to your Pen from any stylesheet on the web. Just put a URL to it here and we'll apply it, in the order you have them, before the CSS in the Pen itself. You can also link to another Pen here (use the .css URL Extension ) and we'll pull the CSS from that Pen and include it.
To work with CSS counters we will use the following properties: counter-reset - Creates or resets a counter. counter-increment - Increments a counter value. content - Inserts generated content. counter() or counters() function - Adds the value of a counter to an element. To use a CSS counter, it must first be created with counter-reset.
The White House announced a handful of additional actions Tuesday intended to curb antisemitism on college campuses and elsewhere amid a rise in discrimination toward Jewish people following the ...
The Online Writing Lab at Purdue University houses writing resources and instructional material, and we provide these as a free service of the Writing Lab at Purdue.
Other videos posted online show the larger crowd, about 200 people, surrounding the protesters and shouting. Counter protesters sung "The Star-Spangled Banner" to drown out pro-Palestinian ...
The trip will also see Xi visit Serbia and Hungary, with the leader's visit to Belgrade coinciding with the 25th anniversary of NATO's bombing of the Chinese embassy in the city that killed three.
Alien: Romulus: Directed by Fede Alvarez. With Cailee Spaeny, Isabela Merced, Archie Renaux, David Jonsson. While scavenging the deep ends of a derelict space station, a group of young space colonizers come face to face with the most terrifying life form in the universe.
Former President Trump said Friday he would be "very proud" to go to jail over violating his gag order imposed by a New York judge in his hush money case. "If anything is mentioned against ...
April 29, 2024—Ottawa—International students enrich Canada's social, cultural and economic fabric.That is why, in recent months, Immigration, Refugees and Citizenship Canada has introduced reforms to the International Student Program, to ensure system integrity while protecting students from fraud and financial vulnerability.
Targeted amendments to modernize the CSIS Act would better equip the Government of Canada to build resilience and to counter the modern threats Canada faces today, including foreign interference. To ensure the safety, security, and prosperity of Canada, CSIS must be able to: Equip national security partners